How to Get the Author information Outside Loop in WP?
The example of Author Box can be seen in the image below;
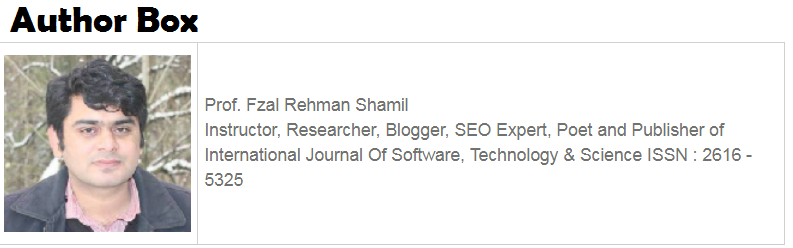
Method 1
This method uses the WP API. This method is the best way of getting author information when you want to get it outside of the Loop.
1 2 3 4 5 6 7 |
<h3>Posts by: <?php the_post(); // queue first post echo get_the_author(); // echo author rewind_posts(); // rewind the loop ?> </h3> |
This source code can be converted into a simpler form. Let’s see it;
1 |
<h3>Posts by: <?php the_post(); echo get_the_author(); rewind_posts(); ?></h3> |
This code displays the author’s Display Name only. If you want to get the entire WP_User object, then you can use the following code:
1 2 3 4 5 6 |
<?php the_post(); // queue first post $author_id = get_the_author_meta('ID'); $curauth = get_user_by('id', $author_id); rewind_posts(); // rewind the loop ?> |
Further, if you want to see other details about the author, then you can call any method of WP_User to get whatever author information you want. Let’s see its code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 |
<div id="archive-view-author"> <?php the_post(); // queue first post $author_id = get_the_author_meta('ID'); $curauth = get_user_by('ID', $author_id); $user_nicename = $curauth->user_nicename; $display_name = $curauth->display_name; $user_description = $curauth->user_description; $user_email = $curauth->user_email; $user_url = $curauth->user_url; $user_website = $curauth->website_name; $user_twitter = $curauth->twitter; rewind_posts(); // rewind the loop ?> <h3>Author Profile: <a href="<?php echo get_author_posts_url($author_id, $user_nicename); ?>"><?php echo $display_name; ?></a></h3> <?php if ($user_description) echo '<p>'. $user_description .'</p>'; ?> <?php if ($user_email) echo get_avatar($user_email, '100'); ?> <?php if ($user_url || $user_twitter) : ?> <ul> <?php if ($user_url) : ?> <li>Website: <a href="<?php echo $user_url; ?>"><?php if ($user_website) : echo $user_website; else : echo $user_url; endif; ?></a></li> <?php endif; ?> <?php if ($user_twitter) : ?> <li>Twitter: <a href="https://twitter.com/<?php echo $user_twitter; ?>">@<?php echo $user_twitter; ?></a></li> <?php endif; ?> </ul> <?php endif; ?> </div> |
Now, you are able to get any author or any user information, even outside of the WordPress Loop.