A program that takes units as input from the user, and unit rates are assigned by the programmer and the program calculates the electricity bill.
Solution: How many values need to be stored in a variable? There are two different rates for each customer. The high rate is for customers with fewer units consumed and low rate is for customers with low units consumed. So we require 2 variables;- rate1 and rate2
- int rate1 and rate2 mean that these two variables only stores integer values.
- When the user inputs the units, then we need to store the units in a unit variable.
- When a total bill is calculated, then we need to store the bill in a variable bill.
Electricity Bill program in c++ using if else
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
#include<iostream> using namespace std; int main() { int rate1, rate2, units, bill; rate1=5; rate2=10; cout<<"enter units consumed by cient:"<<endl; cin>>units; if(units<250) { bill=units*rate1; cout<<"totoal bill is"<<bill<<endl; } else { bill=units*rate2; cout<<"totoal bill is"<<bill<<endl; } } |
Output |
enter units consumed by the client 20 Total bill is = 100 |
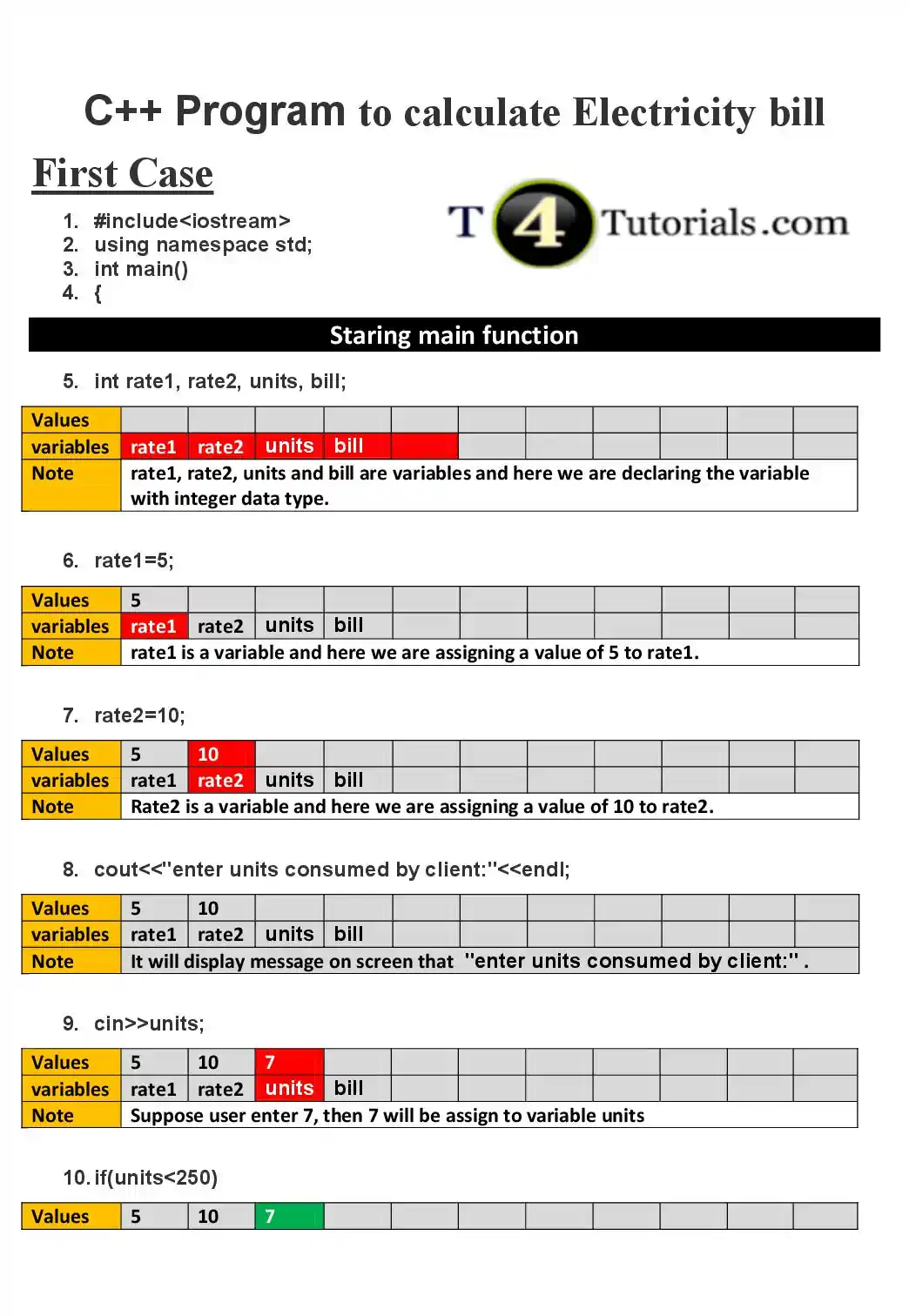
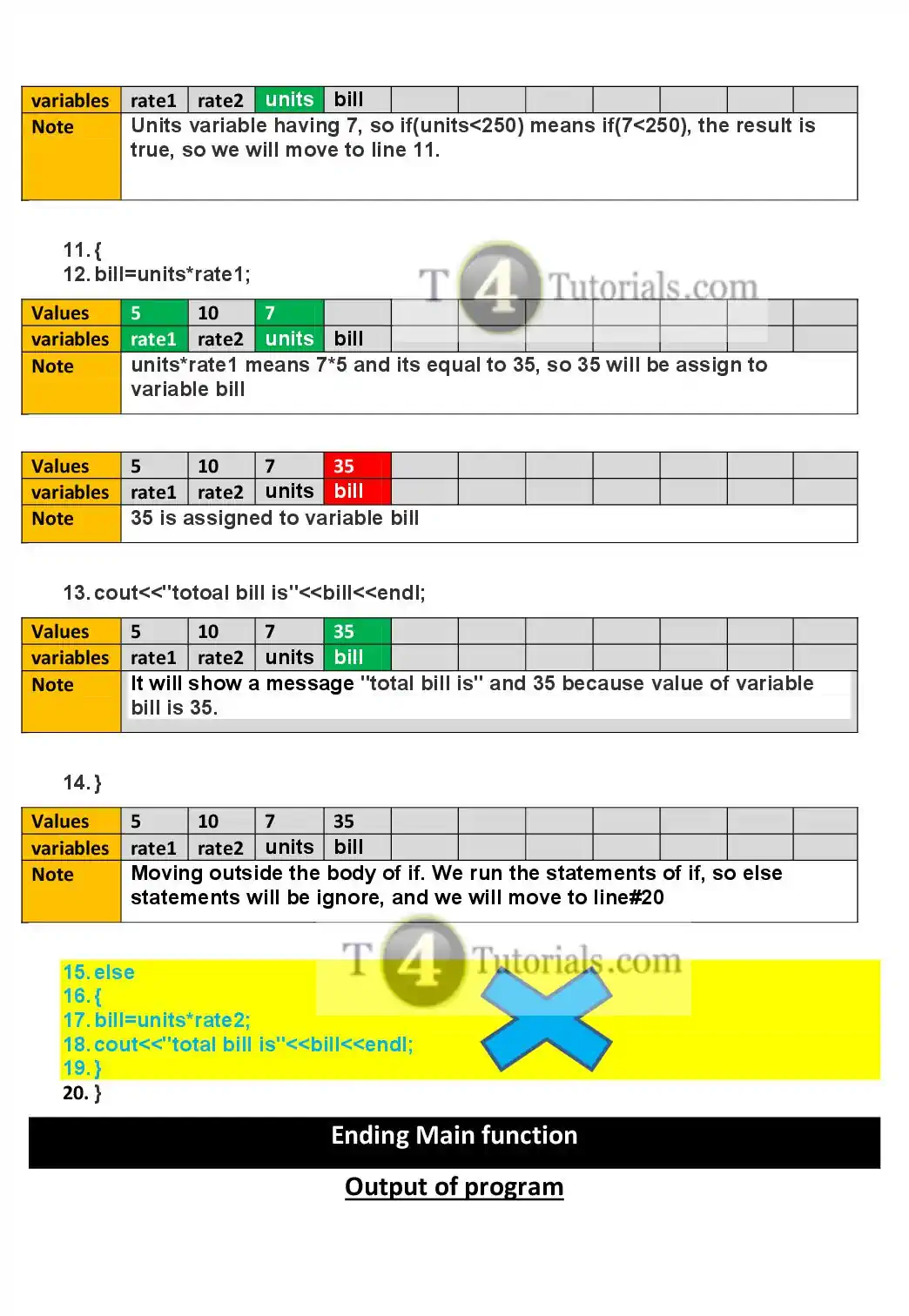
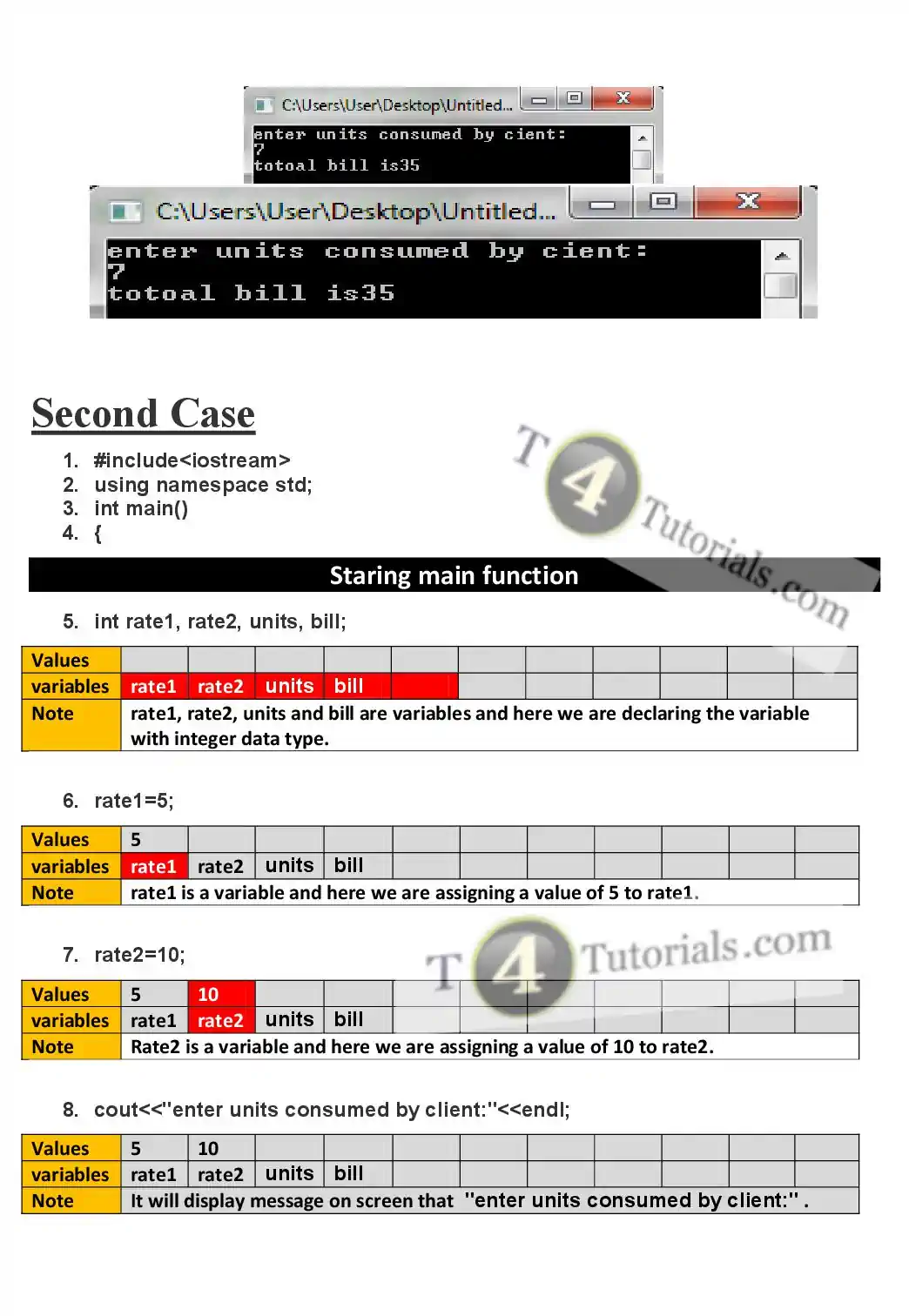
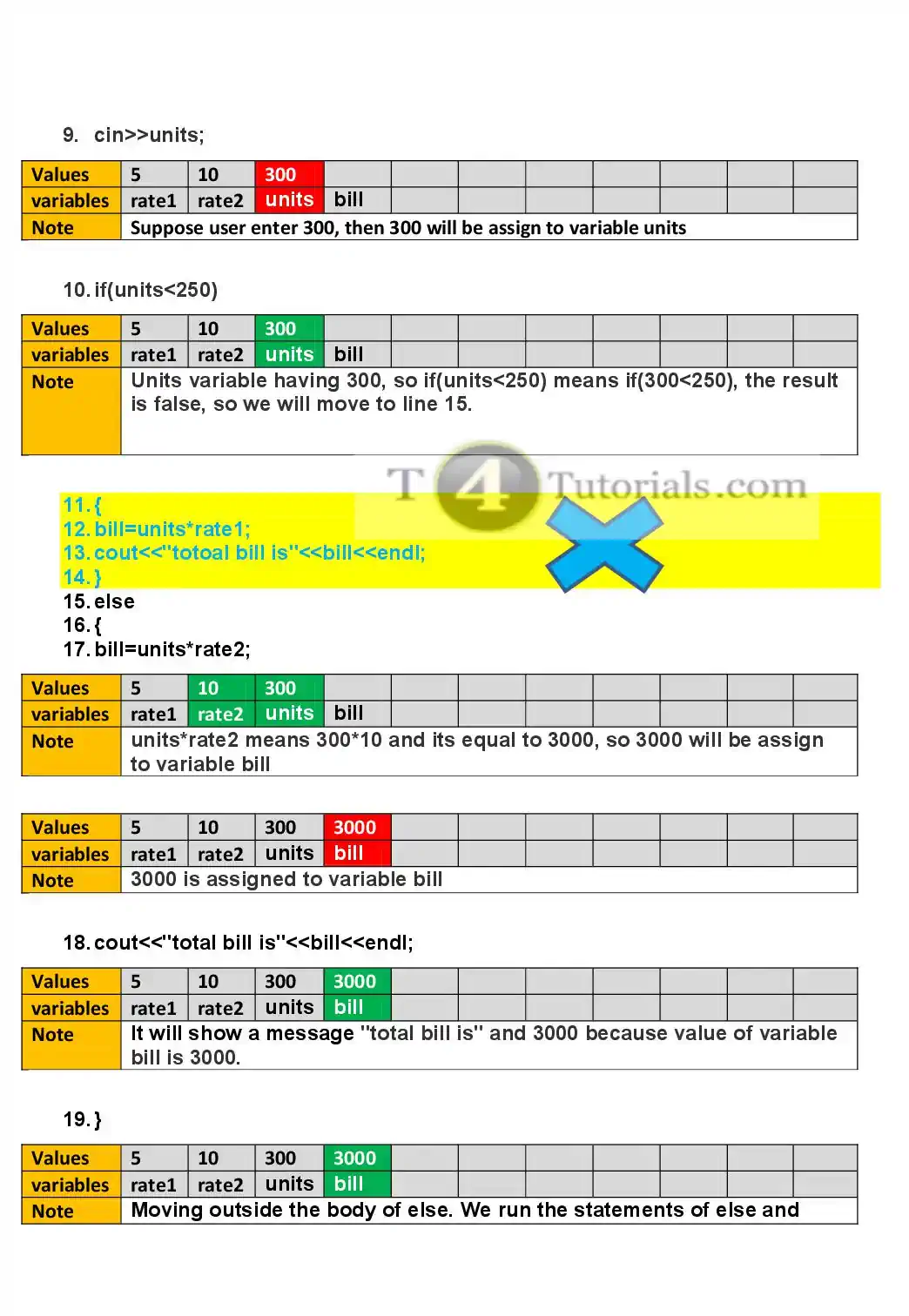
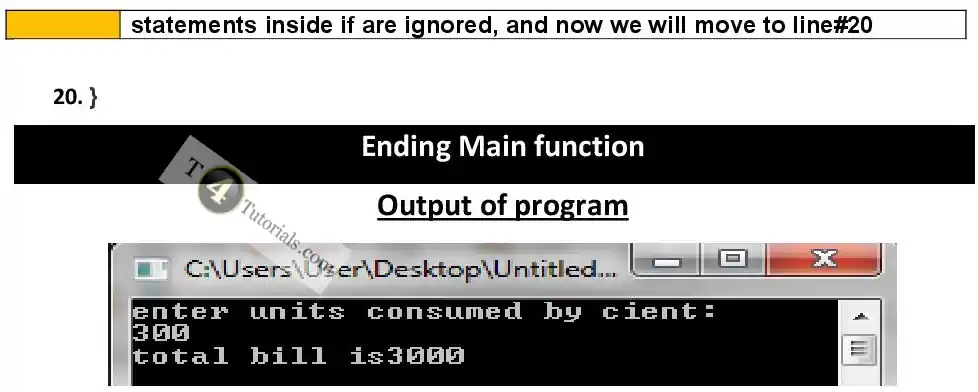
Write a C program to calculate the total electricity bill switch statement
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
#include<iostream> using namespace std; int main() { int Electricity_Unit; float Initial_Amount,Need_To_Pay,s_charge; cout<<"enter the number of Electricity_Units you consumed"<<endl; cin>>Electricity_Unit; switch(Electricity_Unit<=50) { case 1: Initial_Amount=Electricity_Unit*0.50; break; case 0: switch(Electricity_Unit<=150) { case 1: Initial_Amount=25+(Electricity_Unit-50)*0.75; break; case 0: switch(Electricity_Unit<=250) { case 1: Initial_Amount=100+(Electricity_Unit-150)*1.20 ; break; case 0: Initial_Amount=220+(Electricity_Unit-250)*1.50; break; } break; } break; } s_charge=Initial_Amount*0.20; Need_To_Pay=Initial_Amount+s_charge; cout<<"your total bill is Rs"<<Need_To_Pay; } |
C++ Exercise | If else Statement
- calculate the bill
- character is small, capital or a special character
- a number is even or odd
- 0 is a positive or negative number
- a positive and negative number
- Enter Range of numbers and replaced them
- a greater number among three numbers
- Armstrong Number
- ASCII code
- Find the Maximum value program in C++ (C Plus Plus).
- maximum number
- Maximum Number between two numbers
- Student Grade
- the number is divisible by 11 or 5 or not
- Triangle
- a triangle is an equilateral, isosceles or scalene
- Leap year
- character is an alphabet or not
- Grade Percentage
- character is an alphabet, digit, or special character
- character is an uppercase or lowercase.
- Weekdays
- a prime or composite number
- hours and minutes as AM or PM
- swap the values of two numbers
- update even to odd
- Profit Loss
- centimeter into meter and kilometer
- Triangle
- Salary
- Even odd with goto statement.
- area of the circle
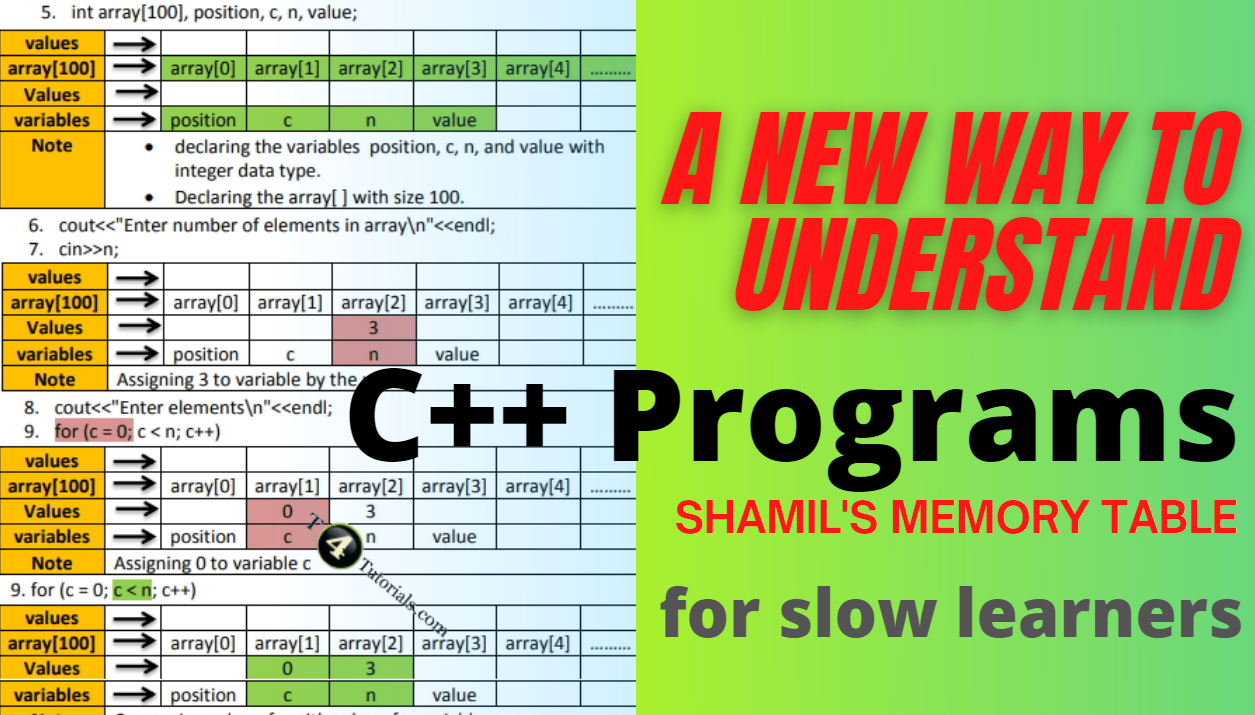