Positive and Negative Number program in PHP
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<?php $number=4; echo "Enter any number: "; echo $number; echo "<br>"; if($number<0) { echo "Number is negative"; } else { echo "Number is positive"; } ?> |
Positive and Negative Number program in PHP with form values entered by the user
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<form method="post"> input number : <br> <input type="number" name="num" /><br><br> <input type="submit" name="submit" value="check number is +ive or -ive "> </form> <?php if(isset($_POST['submit'])) { $number = $_POST['num']; echo $number; echo "<br>"; if($number<0) { echo "Number is negative"; } else { echo "Number is positive"; } } ?> |
Positive and Negative Number program in PHP with database
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
<?php // Create connection $conn = new mysqli('localhost', 'root', '', 't4tutorials.com'); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } echo "Connected To database successfully <br><br>"; ?> <form method="post"> input number : <br> <input type="number" name="num" /><br><br> <input type="submit" name="submit" value="check number is +ive or -ive "> </form> <?php if(isset($_POST['submit'])) { $number = $_POST['num']; echo $number; echo "<br>"; if($number<0) { $h = " negative"; echo $h; } else { $h = " positive"; echo $h; } $sql = "INSERT INTO `tableh` (`Number`, `+ive / -ive`) VALUES ('$number', '$h')"; if ($conn->query($sql) === TRUE) { echo "<br> Record Added successfully"; } else { echo "Error: " . $sql . "<br>" . $conn->error; } } ?> |
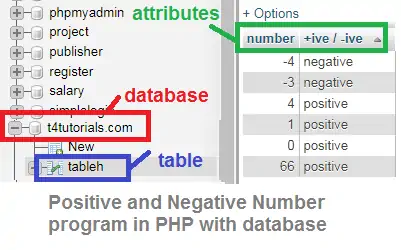
PHP Program to Separate Positive & Negative Numbers
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
<?php $myarr = array(12,-14,-59,90,100); //now loop through the array and separate foreach($myarr as $num){ if($num > 0){ $positive[] = $num; }else{ $negative[] = $num; } } //print both positive and negative array values print_r($positive); print_r($negative); ?> |
Output
Array ( [0] => 12 [1] => 90 [2] => 100 ) Array ( [0] => -14 [1] => -59 )PHP Program to Separate Positive & Negative Numbers with form
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
<html> <head> <title>Separate Positive & Negative numbers in an Array in PHP</title> </head> <body> <h3>PHP Program to Separate Positive & Negative </h3> Enter the Numbers separated by Commas <br /> (eg: 12,-23,56,-14,-25,10) <br /><br /> <form method="post"> <input type="text" name="numbers"/> <button type="submit">Separate</button> </form> </body> </html> <?php if($_POST) { //get the post value from form $numbers = $_POST['numbers']; if($numbers != ''){ //separate the numbers and make into array $numArray = explode(',', $numbers); //now this array has both positive and negative integers //let's separate it foreach($numArray as $num){ if($num > 0){ $positiveArr[] = $num; }else{ $negativeArr[] = $num; } } print "Positive Values: ". print_r($positiveArr,true)."<br>"; print "Negative Values: ". print_r($negativeArr,true); } } ?> |