Simple PHP Program to count the vowels, digits, and spaces in a string
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
<html> <body> <div> <h1>program for counting vowal,digits.spaces in string</h2> <?php { $string="ali is good boy222"; $vowels = array('a','e','i','o','u'); $len = strlen($string); $num = 0; for($i=0; $i<$len; $i++){ if(in_array($string[$i], $vowels)) { $num++; } echo "$num"; } function countDigits( $string ) { return preg_match_all( "/[0-9]/", $string ); } substr_count($string, ' '); echo "Number of vowels : <span style='color:green; font-weight:bold;'>". $num."</span>"; echo "<br>"; echo "Number of digits :".countDigits($string); echo "<br>"; echo "Number of spaces :" . substr_count($string," "); } ?> </body> </html> |
Simple PHP Program to count the vowels, digits, and spaces in a string using while loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
<html> <body> <form action="" method="post"> <input type="text" name="string" /> <input type="submit" /> </form> </body> </html> <?php if($_POST) { $string = strtolower($_POST['string']); $vowels = array('a','e','i','o','u'); $len = strlen($string); $num = 0; $i=0; while( $i<$len){ if(in_array($string[$i], $vowels)) { $num++; } $i++; } function countDigits( $string ) { return preg_match_all( "/[0-9]/", $string ); } substr_count($string, ' '); echo "Number of vowels : <span style='color:green; font-weight:bold;'>". $num."</span>"; echo "<br>"; echo "Number of digits :".countDigits($string); echo "<br>"; echo "Number of spaces :" . substr_count($string," "); } ?> |
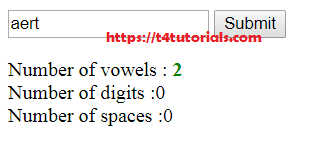
PHP Program to count the vowels, digits, and spaces in a string with the form
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
<html> <body> <form action="" method="post"> <input type="text" name="string" /> <input type="submit" /> </form> </body> </html> <?php if($_POST) { $string = strtolower($_POST['string']); $vowels = array('a','e','i','o','u'); $len = strlen($string); $num = 0; for($i=0; $i<$len; $i++){ if(in_array($string[$i], $vowels)) { $num++; } } function countDigits( $string ) { return preg_match_all( "/[0-9]/", $string ); } substr_count($string, ' '); echo "Number of vowels : <span style='color:green; font-weight:bold;'>". $num."</span>"; echo "<br>"; echo "Number of digits :".countDigits($string); echo "<br>"; echo "Number of spaces :" . substr_count($string," "); } ?> |