Optimal Page replacement Memory Management Operating Systems OS
According to Optimal Page replacement OS replaces the page from the memory that is not used for a long period of time.
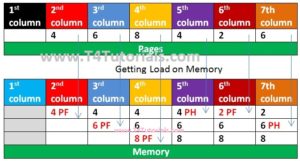
PF stands for a page fault Read More . FH is used in a table to represent the page hit. Read More
1st column: First of all, all memory is free.
2nd column: Page 4 is loaded on memory. A page fault Read More occurs because page 4 is already not on memory.
3rd column: Page 6 is loaded on memory. A page fault occurs.
4th column: Page 8 is loaded on memory. A page fault occurs.
5th column: Page 4 is already loaded on memory. No page fault.
6th column: Page 2 is loaded on memory. A page fault occurs.
7th column: Page 6 is already loaded on memory. No page fault. Read More
C++ program to demonstrate the Optimal Page Replacement Algorithm
// CPP program for optimal page page replacement algorithm in operating systems #include <bits/stdc++.h> using namespace std; // Page exists in memory or not? bool PageSearch(int number, vector<int>& Frames) { for (int loop = 0; loop < Frames.size();loop ++) if (Frames[loop] == number) return true; return false; } // Fins unused Frames int predict(int page[], vector<int>& Frames, int pn, int index) { int res = -1, farthest = index; for (int loop = 0; loop < Frames.size();loop ++) { int loop2; for (loop2 = index; loop2 < pn; loop2++) { if (Frames[loop] == page[loop2]) { if (loop2 > farthest) { farthest = loop2; res = loop; } break; } } // Suppose the page is never referenced in future, if (loop2 == pn) return loop; } // If all of the Frames were not in future, // return any of them, we return 0. Otherwise // we return res. return (res == -1) ? 0 : res; } void optimalAlgorithm(int page[], int pn, int TotalFrames) { vector<int> Frames; // Traverse array with page reference array to check for the PageHit and miss. int PageHit = 0; for (int loop = 0; loop < pn; loop++) { // Page detected in memory if (PageSearch(page[loop], Frames)) { PageHit++; continue; } // Page not detected in memory if (Frames.size() < TotalFrames) Frames.push_back(page[loop]); // Find the page to be replaced. else { int loop2 = predict(page, Frames, pn, loop + 1); Frames[loop2] = page[loop]; } } cout << " Total Page misses = " << pn - PageHit << endl; cout << "Total Page Hits = " << PageHit << endl; } int main() { int page[] = { 4, 6, 8, 4, 2, 6 }; int pn = sizeof(page) / sizeof(page[0]); int TotalFrames = 3; optimalAlgorithm(page, pn, TotalFrames); return 0; }
Output
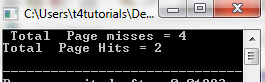