PHP Program to find the area of a rectangle with form and database
In this tutorial, we will learn about the followings.- PHP Program to find the area of rectangle
- PHP Program to find the area of rectangle with form
- PHP Program to find the area of rectangle with database
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<!doctype html> <html> <head> <meta charset="utf-8"> <title>Untitled Document</title> </head> <body> <?php $length = 14; $width = 12; echo "area of rectangle is $length * $width= " . ($length * $width) . "<br />"; echo "perimeter of rectangle is $length * $width= " . (2*($length+$width)) . "<br />"; ?> </body> </html> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
<form method="POST"> Enter the length <input type = "text" name = "le"> <br/> Enter the breadth <input type = "text" name = "br"> <br/> <input type= "submit" value = "submit" name="btnsubmit"> </form> <?php if(isset($_POST['btnsubmit'])) { $l=$_POST['le']; $b=$_POST['br']; $area=$l*$b; $peri= 2*($l+$b); echo "The aea of rectangle ".$area."<br/>"; echo "The perimeter of rectangle ".$peri."<br/>"; } ?> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 |
<!doctype html> <html> <head> <meta charset="utf-8"> <title>Untitled Document</title> </head> <body> <form method="POST"> Enter the length <input type = "text" name = "le"> <br/> Enter the breadth <input type = "text" name = "br"> <br/> <input type = "submit" name="btnsubmit" value = "submit"> </form> <?php $server = "localhost"; $user="root"; $password=""; $database="RectangleArea"; $con=mysqli_connect("localhost","root","","RectangleArea"); if(isset($_POST['btnsubmit'])) { $l=$_POST['le']; $b=$_POST['br']; $area=$l*$b; $peri= 2*($l+$b); if(isset($_POST['btnsubmit'])) { $res= mysqli_query($con,"INSERT INTO rectangle (lenghth,breadth,area ,perimeter ) VALUES ('$l','$b','$area','$peri')"); } echo "The aea of rectangle ".$area."<br/>"; echo "The perimeter of rectangle ".$peri."<br/>"; } ?> </body> </html> |
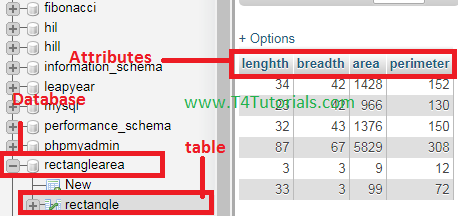