Inserting Multiple Rows at the same time into a Table in PHP with Procedural method
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
<?php $T4TutorialsDotCom = mysqli_connect("localhost", "root", "", "t4tutorials"); // Check connection if($T4TutorialsDotCom === false){ die("ERROR: Could not connect. " . mysqli_connect_error()); } // Attempt insert query execution $sql = "INSERT INTO persons (first_name, last_name, email) VALUES ('ali', 'khan', '[email protected]'), ('akram', 'niazi', '[email protected]'), ('asad', 'cheema', '[email protected]'), ('arshad', 'khokhar', '[email protected]')"; if(mysqli_query($T4TutorialsDotCom, $sql)){ echo "Records added successfully."; } else{ echo "ERROR: Could not able to execute $sql. " . mysqli_error($T4TutorialsDotCom); } // Close connection mysqli_close($T4TutorialsDotCom); ?> |
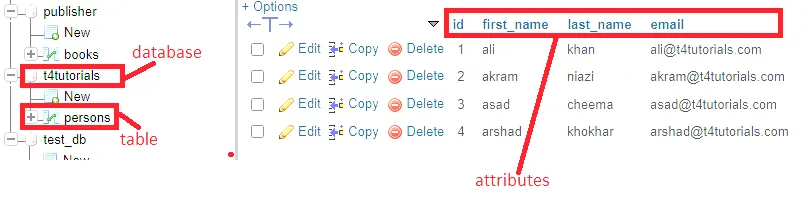
Inserting Multiple Rows at the same time into a Table in PHP with Object Oriented method
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
<?php $mysqli = new mysqli("localhost", "root", "", "t4tutorials"); // Check connection if($mysqli === false){ die("ERROR: Could not connect. " . $mysqli->connect_error); } // Attempt insert query execution $sql = "INSERT INTO persons (first_name, last_name, email) VALUES ('ali', 'khan', '[email protected]'), ('akram', 'niazi', '[email protected]'), ('asad', 'cheema', '[email protected]'), ('arshad', 'khokhar', '[email protected]')"; if($mysqli->query($sql) === true){ echo "Records inserted successfully."; } else{ echo "ERROR: Could not able to execute $sql. " . $mysqli->error; } // Close connection $mysqli->close(); ?> |
Inserting Multiple Rows at the same time into a Table in PHP with PDO method
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
<?php try{ $pdo = new PDO("mysql:host=localhost;dbname=t4tutorials", "root", ""); // Set the PDO error mode to exception $pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); } catch(PDOException $e){ die("ERROR: Could not connect. " . $e->getMessage()); } // Attempt insert query execution try{ $sql = "INSERT INTO persons (first_name, last_name, email) VALUES ('ali', 'khan', '[email protected]'), ('akram', 'niazi', '[email protected]'), ('asad', 'cheema', '[email protected]'), $pdo->exec($sql); echo "Records inserted successfully."; } catch(PDOException $e){ die("ERROR: Could not able to execute $sql. " . $e->getMessage()); } // Close connection unset($pdo); ?> |
Download Full code with database