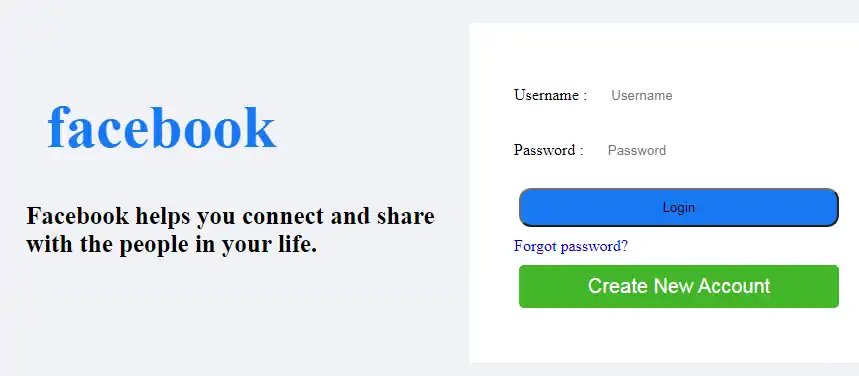
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 |
<?php include "config.php"; if(isset($_POST['but_submit'])){ $uname = $_POST['txt_uname']; $password = $_POST['txt_pwd']; if ($uname != "" && $password != ""){ $sql_query = "select count(*) as cntUser from users where username='".$uname."' and password='".$password."'"; $result = mysqli_query($con,$sql_query); $row = mysqli_fetch_array($result); $count = $row['cntUser']; if($count > 0){ $_SESSION['uname'] = $uname; header('Location: home.php'); }else{ echo "Invalid username and password"; } } } ?> <html> <head> <title>facebook</title> </head> <style> body { background-color:#f0f2f5; } .a{ color:#1877f2; font-size:60; margin-top:200px; margin-left:160px; font-style: bold; } .b{ padding: 0px 0px 20px; color:black; font-size:25; margin-top:15px; margin-left:140px; margin-right:55%; } button { background-color:steelblue; width: 100%; color:white; padding: 15px; margin: 10px 0px; border: none; cursor: pointer; border-radius: 5px; background-color: #1877f2; font-size: 20px; } form { border: 3px solid #f1f1f1; } input[type=text], input[type=password] { width: 60%; margin: 8px 0; padding: 12px 20px; display: inline-block; border: 1px grey; box-sizing: 25%; } button:hover { opacity: 0.7; } .cancelbtn { width: 80%; padding: 10px 18px; margin: 10px 5px; background-color: #42b72a; text-align: center; text-decoration:none; } .login{ background-color: #1877f2; text-align: center; width: 80%; padding: 10px 18px; margin: 10px 5px; text-align: center; border-radius: 12px; } .container { padding: 45px; background-color:white; width:400px; position: absolute; float: right; right: 100px; top: 20%; } </style> <body> <h1 class='a'> facebook<h1> <h2 class='b'>Facebook helps you connect and share with the people in your life.</h2> <form method="post" action=""> <div class="container" id="div_login"> <label>Username : </label> <input type="text" class="textbox" id="txt_uname" name="txt_uname" placeholder="Username" /> <br><label>Password : </label> <input type="password" class="textbox" id="txt_uname" name="txt_pwd" placeholder="Password"/><br> <input class="login" type="submit" value="Login" name="but_submit" id="but_submit" /> <br> <a style="text-decoration: none;" href="#">Forgot password? </a> <br> <button onClick="document.location='signup.php'" type="button" class="cancelbtn">Create New Account </button><br> </div> </form> </body> </html> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
<?php include "config.php"; // Check user login or not if(!isset($_SESSION['uname'])){ header('Location: index.php'); } // logout if(isset($_POST['but_logout'])){ session_destroy(); header('Location: index.php'); } ?> <!doctype html> <html> <head></head> <body> <h1>Homepage</h1> <form method='post' action=""> <input type="submit" value="Logout" name="but_logout"> </form> </body> </html> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<?php session_start(); $host = "localhost"; /* Host name */ $user = "root"; /* User */ $password = ""; /* Password */ $dbname = "tutorial"; /* Database name */ $con = mysqli_connect($host, $user, $password,$dbname); // Check connection if (!$con) { die("Connection failed: " . mysqli_connect_error()); } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 |
<?php include('dbcon.php'); error_reporting(0); ?> <html> <html lang="en"> <head> <link rel="stylesheet" type="text/css" href="style.css"> </head> <style> .form-wrapper { width:400px; height:550px; position: absolute; top: 50%; left: 48%; margin: -184px 0px 0px -155px; background: white; padding: 15px 25px; border-radius: 5px; } body { color: #fff; font: 87.5%/1.5em 'Open Sans', sans-serif; background:url(img/pic2.png)no-repeat center center fixed; -webkit-background-size: cover; -moz-background-size: cover; -o-background-size: cover; background-size: cover;} .form-item { width:45%; float: left; background: lightgreen; } .form-item input{ border: none; background:transparent; color: #fff; margin-top:11px; font-family: 'Open Sans', sans-serif; font-size: 1.2em; height: 40px; padding: 0 16px; width: 100%; padding-left: 55px; } .form-item2 { width:45%; float: left; background:aqua; margin-left: 5px; } .form-item2 input{ border: none; background:transparent; color: #fff; margin-top:11px; font-family: 'Open Sans', sans-serif; font-size: 1.2em; height: 40px; padding: 0 16px; width: 100%; padding-left: 55px; margin-left: 10px; } </style> <body> <div class="form-wrapper"> <h3>Sign up</h3> <p style="color: black">it'a quick and easy</p> <div class="reminder"> <br> <form method ="GET" > <div class="form-item"> <input type="text" name = "fname" placeholder = "First name"> </div> <div class="form-item2"> <input type="text" name = "lname" placeholder = "Last Name "> </div> <div> <input type="text" name="Username" placeholder="Username"> </div> <div> <input type="text" name="password" placeholder="Password"> </div> <div class="button-panel"> <input type="submit" class="button" name = "submit" > </div> </form> <?php if($_GET['submit']) { $Id = $_GET['id']; $firstName = $_GET['fname']; $lastname = $_GET['Lname']; $username = $_GET['Username']; $password = $_GET['password']; if($Id != ""&&$firstName != ""&& $lastname != ""&& $password != ""&& $username != "") { $query = "INSERT INTO users VALUES('$Id','$firstName','$lastname','$username','$password')"; $data= mysqli_query($con,$query); if($data) { echo "data is inserted"; } else { echo "Enter All data"; } } } ?> <p><a href="Index.php">click hare to login </a></p> </div> </div> </body> </html> |