Diamond Shape Program Code in PHP
In this tutorial, we learn the code of diamond shape program in PHP.Diamond Shape Program Code in PHP
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
<html> <head> <title>Diamond Shape!</title> </head> <body> <?php $limit = 10; $space = $limit; for ($i = 1; $i <= $limit; $i++) { for ($j = 1; $j <= $space; $j++) { echo "&nbsp;&nbsp;"; } $space--; for ($j = 1; $j <= 2 * $i - 1; $j++) { echo "*"; } echo "<br>"; } $space = 2; for ($i = 1; $i <= $limit; $i++) { for ($j = 1; $j <= $space; $j++) { echo "&nbsp;&nbsp;"; } $space++; for ($j = 1; $j <= 2 * ($limit - $i) - 1; $j++) { echo "*"; } echo "<br>"; } ?> </body> </html> |
Diamond Shape in PHP with Form Values entered by the user
In this tutorial, we learn the code of diamond shape program in PHP with form values entered by the user.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
<html> <head> <title>print Diamond Shape!</title> </head> <body> <h2>print Diamond Shape!</h2> <form method="post"> Enter the limit: <input type="number" name="limit" min="2" max="100" />&nbsp;<input type="submit" value="Print Diamond Pattern!" name="print" /> </form> </body> </html> <?php //check if the form is submitted $con = mysqli_connect("localhost","root","","mydatabase"); //echo $con; // Check connection if (mysqli_connect_errno()) { echo "Failed to connect to MySQL: " . mysqli_connect_error(); } if (isset($_POST['print']) && $_POST['print']) { $limit = $_POST['limit']; $sql = "INSERT INTO diamond (DiamondValue) VALUES ($limit)"; if ($con->query($sql) === TRUE) { echo "New record created successfully"; echo "<br>"; } else { echo "Error: " . $sql . "<br>" . $con->error; } $space = $limit; for ($i = 1; $i <= $limit; $i++) { for ($j = 1; $j <= $space; $j++) { echo "&nbsp;&nbsp;"; } $space--; for ($j = 1; $j <= 2 * $i - 1; $j++) { echo "*"; } echo "<br>"; } $space = 2; for ($i = 1; $i <= $limit; $i++) { for ($j = 1; $j <= $space; $j++) { echo "&nbsp;&nbsp;"; } $space++; for ($j = 1; $j <= 2 * ($limit - $i) - 1; $j++) { echo "*"; } echo "<br>"; } } ?> |
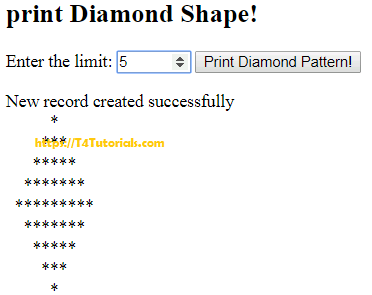
Database View for Diamond Shape Program
