Javascript Tutorials and Programs
Javascript Tutorials and Programs are the topics of discussion for today tutorial.
Addition, Subtraction, Multiplication, and Division of two numbers
Algorithm of the sum of two numbers
Step 1: Start
Step 2: Declare variables number1, number2 and sum.
Step 3: Input values number1 and number2.
Step 4: Add number1 and number2 and assign the result to sum.
Sum = number 1 + number2
Step 5: Display sum
Pseudocode of the sum of two numbers
Begin
Entering number1
Entering number2
Compute sum as number1 and number2
Display sum of given two numbers in the sum
End
Program of two numbers
1 2 3 4 5 6 7 |
function add(){ var firstnumber,secondnumber,sum; firstnumber = parseInt(prompt("enter first value")); secondnumber = parseInt(prompt("enter second value")); sum = firstnumber + secondnumber; document.write("the sum of two numbers is",sum); } |
Program of Multiplying two numbers
1 2 3 4 5 6 7 |
function mul(){ var firstnumber,secondnumber,multi; firstnumber = parseInt(prompt("enter first value")); secondnumber = parseInt(prompt("enter second value")); multi = firstnumber * secondnumber; document.write("the sum of two numbers is",multi); } |
Program of Division of two numbers
1 2 3 4 5 6 7 |
function divide(){ var firstnumber,secondnumber,div; firstnumber = parseInt(prompt("enter first value")); secondnumber = pasrseInt(prompt("enter second value")); div = firstnumber / secondnumber; document.write("the sum of two numbers is",div); } |
Program of Subtraction of two numbers
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
<html> <head> <script> function subtract(){ var firstnumber,secondnumber,sub; firstnumber = parseInt(prompt("enter first value")); secondnumber = parseInt(prompt("enter second value")); sub = firstnumber - secondnumber; document.write("the sum of two numbers is",sub); } </script> </head> <body onload = "subtract()"> </body> </html> |
Addition of Three Numbers
Algorithm of Addition of Three Numbers in Javascript
- Start
- First of all, we open the specific programming software to solve the program.
- Then open the new file and save file name of your choice.
- Start program with HTML open syntax
- Then JavaScript open syntax.
- Declare the function name in this program I declare (Calculate) function name.
- Then declare the variables name.
- Then the addition of values assigns and stores the equal numbers in n4.
- The value of button assign through which data is displayed.
- After that close the script syntax.
- Close the head syntax.
- Open the body syntax.
- Open the form syntax and assign the name of a form of your choice.
- I assign the (frm) name to form.
- Now we move to the front end of the program.
- Using HTML we use input data type in which user enter the values to sum.
- Using three input data type.
- We use the button through which data is added and display the sum of the numbers in button.
Addition of Three Numbers in Javascript
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
<html> <head> <script> function Calculate(n1,n2,n3,n4) { var n1=parseFloat(n1) var n2=parseFloat(n2) var n3=parseFloat(n3) var n4=n1+n2+n3 frm1.txt5.value=n4 } </script> </head> <body> <form name="frm1"> Enter 1stNumber: <input name="txt1" type="text" /> <br> <br> Enter 2nd Number: <input name="txt2" type="text" /> <br> <br> Enter 3rd Number: <input name="txt3" type="text" /> <br> <br> Sum Of Numbers=> <input name="txt5" type="button" value="Add" onclick="Calculate(txt1.value,txt2.value,txt3.value)"> </form> </body> </html> |
Output
The greatest number among 3 numbers in Javascript
Algorithm of greatest number among 3 numbers
Step1: Start.
Step2: Declare three variables x,y,z.
Step3: Get a number from the user or already give value to these variables.
Step4: If(x>y)
If(x>z)
Display x is the greatest number
Else
Display z is the greatest number
If(y>z)
Display y is the greatest number
Else
Display z is the greatest number
Step 5: End
Program explanation
- Take the three numbers and store it in the variables x, y, and z respectively.
- Firstly check if the x is greater than y.
- If it is, then prints the output as “x is the greatest among three”.\
- Otherwise, print the output as “z is the greatest among three”.
- If the x is not greater than y, then check if y is greater than z.
- If it is, then prints the output as “y is the greatest among three”.
- Otherwise, print the output as “z is the greatest among three”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 |
<html> //starting html <head> // starting head <script> // starting java_script function largest() //function of java script { var no1; //initialize variable var no2; //initialize variable var no3; //initialize variable no1= Number(document.getElementById("X").value); //get value by id no2 = Number(document.getElementById("Y").value); //get value by id no3 = Number(document.getElementById("Z").value); //get value by id if(no1>no2 && no1>no3) //if condition { window.alert(no1+"-is greatest"); // display greater number } else if(no2>no1 && no2>no3) //else if { window.alert(no2+"-is greatst"); // display greater number } else if(no3>no1 && no3>no1) //else if { window.alert(no3+"is greatest"); // display greater number } } </script> //java script end </head> //head end <body> //body start <h1>Calculate largest among three numbers</h1> //heading <hr color="cyan"> //heading color <br> //next line Enter number 1: <input type="text" id="X"></input><br> //textbox Enter number 2: <input type="text" id="Y"></input><br> Enter number 3: <input type="text" id="Z"></input><br> <hr color="cyan"> <center><button onclick="largest()">OK</button> //button </body> //body start </html> |
Output
Algorithm of Number of vowels digits consonant and spaces in the string (Javascript)
1 2 3 4 5 6 7 8 9 |
Algorithm for program Step 1: start with html tag Step 2: write a code for text box Step 3: write code for click button Step 4: used onClick function on button Step 5: Declare variable named “str” to store value gathered value from user Step 6: Declare variable v,c,d Step 7: use replace function and length function to calculate number of vowels digits and consonants. Step 8: display out on screen |
Armstrong Number in Javascript
Algorithm of Armstrong Number
START
Step 1 → Take variable ’NO’
Step 2 → input some value to variable no
Step 3 → MAKE VARIABLE NAME arm and assign zero to it
Step 4 → create another variable name LAST
Step 5 → TAKE mod of no with 10 and store in variable last
Step 6 →take a cube of last and add it to arm
Step 7 →divide no with 10 and store value to no
Step 8→ If Sum equals to Arms print Armstrong Number
Step 9 → If Sum does not equal to Arms print Not Armstrong Number
STOP
Program of Armstrong Number
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
<!DOCTYPE html> <html> <head> <title>Armstrong Number by Waqas 3488</title> </head> <body> <input type="text" id="no" name="numberr"> <input type="button" value="clickhere" onclick="Armstrong()"> <script > function Armstrong() { var no=document.getElementById('no').value; var dup=no; var arm=0; while(no!=0) { var last=no%10; arm+=(last*last*last); no/=10; no=parseInt(no); } if (dup==arm) { alert("Armstrong number"); } else alert("not Armstrong") } </script> </body> </html> |
Output
Checking Time Difference Program in JS
Algorithm of Checking Time Difference
Step 1: Start.
Step 2: Declare Variables as date1, date2, diff, days, hours, minutes and seconds.
Step 3: Read these Variables date1, date2, diff, days, hours, minutes and seconds.
Step 4: Calculate diff, days, hours, minutes and seconds by using Formulas:
diff ← (date1-date2)/1000
days ← (diff/86400)
hours ← (diff/3600) % 24
minutes ← (diff/60) % 60
seconds ← diff % 60
Step 5: Display Time difference b/w two dates in days, hours, minutes and seconds.
Step 6: Stop.
Pseudo Code of Checking Time Difference
- This program checks the time difference b/w two dates in days, hours, minutes and seconds.
- Sets input as date1, date2.
- Read variables diff, days, hours, minutes and seconds.
- Calculate diff of two dates using the formula:
- Diff= date1-date2)/1000
- Calculate days, hours, minutes and seconds using respective formulas.
- Display result/difference.
Program of Checking the Time Difference
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 |
<html> <head> <title> JS to check Time difference b/w two Dates </title> </head> <body> <script> var date1, date2; date1 = new Date( "march 5, 2019 10:15:35" ); document.write(""+date1); date2 = new Date( "march 6, 2019 11:30:20" ); document.write("<br>"+date2); var diff = Math.abs(date1 - date2) / 1000; // days b/w two dates var days = Math.floor(diff / 86400); document.write("<br>Difference of (Days): "+days); // hours b/w two dates var hours = Math.floor(diff / 3600) % 24; document.write("<br>Difference of (Hours): "+hours); // minutes b/w two dates var minutes = Math.floor(diff / 60) % 60; document.write("<br>Difference of (Minutes): "+minutes); // seconds b/w two dates var seconds = diff % 60; document.write("<br>Difference of (Seconds): "+seconds); </script> </body> </html> |
Output
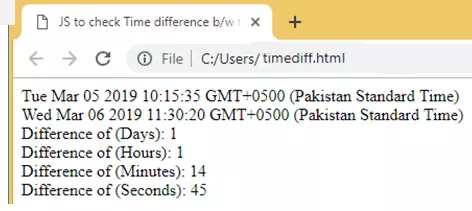
Explanation
First, we are declaring two variables date1 and date2.
Now we are assigning or setting values in those two date variables.
After that, we are declaring variables diff, days, hours, minutes and seconds along with their respective formulas.
The formulas will calculate values as the difference in time in days, hours, minutes and seconds.
It’s time to Display the difference in time using output statements.
Length of number in Javascript
Algorithm of Length of a number in Javascript
- First, make the function to count the length of number and make a button to run behind the button.
- In the next step, they were looking for reference using id and easily selector the specific tag.
- In the script call the function using the value with var no1 12666.
- The document write is used for the expression length.
- And also the .to string which is used to compare the number.
- In the next step, they will get the specified value by using attributes.
Pseudo Code of Length of a number in Javascript
The program is used to count the number of value.
Onclick=” the count()”
Make a function to calculate the length of number. That will count or hold the value of number.
<p Id = “demo”></p>
Looking for the id means to easily select the specific tag. And also look for the reference of which is p=id here is the id which contains the reference.
Function the count{
Here is the value which we want to calculate the length of the number. And there is pre value which is stored in the form of a number
Var no1 = 656554664;
And also number 2 which also contain the value
Var no2 = 145796646;
Document.write (“”) no1.string().lenght
In this value is the value of no1 and is adding and also the to compare the value of the string.
Document.write (“”) no2.string().lenght
In this value is the value of no2 adding and also the to compare the value of the string
Document.getElementById(“demo”).innerHTML = n;
This is the value is specified by using the value of the attribute.
Flowchart of Length of a number in Javascript
Step: 1
Step on the function which is used to count or call.
Step: 2
The function count is used to count the number
Step: 3
Document.Write is used to convert it into a string and pass it to the attributes to show the result
Step: 4
In this step, the number is adding also converting into a string.
Step: 5
In the last step the get value number from to string and recognized by id and show the length of number.
Program of Length of a number in Javascript
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
<html> <body> <button onclick="theCount()">Click Here</button> <p id="demo"></p> <script> function theCount(){ var no1 = 789258693; var no2 = 159963478523; document.write("Length of number "+no1+" = "+ no1.toString().length); document.write("<br>Length of number "+no2+" = "+ no2.toString().length); document.getElementById("demo").innerHTML = n; } </script> </body> </html> |
Output
Length = 789258693 = 9
Length = 159963478623 = 12
Take three variables and store name, address, and phone number
Algorithm of the program to take three variables and store name, address, and phone number
Step 1: Start
Step 2: Declare variables for name address and phone number.
Step 3: Input values for variables.
Step 4: show output
Program to take three variables and store name, address, and phone number
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<html> <head> <script> function info(){ var name,address,phonenumber; name = prompt("enter name"); address = prompt("enter address"); phonenumber = prompt("enter phone number"); document.write("\nMy name is ",name); document.write("\nMy address is ",address); document.write("\nMy phone number is ",phonenumber); } </script> </head> <body onload = "info()"> </body> </html> |
Printing the Highest Value in JavaScript
Flowchart of Printing the Highest Value in JavaScript
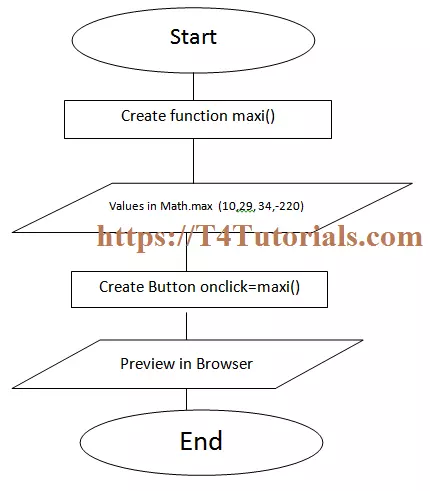
Algorithm of printing the Highest Value in JavaScript
- Open a new HTML file.
- Write Code in the script tag.
- Create a function of finding the maximum value.
- Use built-in function Math.max to find the maximum value.
- Create a button in the HTML body.
- Call function on the onclick button.
- The output will be displayed in the browser.
Program of printing the Highest Value in JavaScript
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
<html> <head> <title>Highest Value </title> </head> <body> <center><h3>Highest value finding Program</h3> <button onclick="maxi()">Click !</button></center> </body> <script language="javascript"> function maxi() { document.write(Math.max(10,29,34,-220)); } </script> </html> |
String length in javascript
Algorithm of finding the string length in javascript
- Open HTML, head and body tags.
- Under head tag, open title tag and set the title of the program and close title tag.
- Underbody tag open script tag.
- In script, the tag makes the object of Str.leanth() and write a string that is to be measured.
- Show output on screen close script tag.
- Close all other open tags.
Program of finding the string length in javascript
1 2 3 4 5 6 7 8 9 10 11 |
<html> <head> <title>JavaScript String length Property</title> </head> <body> <script> var str = new String( "This is string that is to be measured" ); document.write("lesnth of the string is:" + str.length); </script> </body> </html> |
Output
Length of the string is: 37
Area of Rectangle in Javascript
Algorithm of Area of Rectangle in Javascript
- Take the length of the rectangle.
- Then take the width of the rectangle.
- Now multiply length and width of the rectangle that user was given.
- And the answer will display.
Pseudocode of Area of Rectangle in Javascript
area = width x length.
Display “enter the length of your rectangle:”
Input length.
Display “enter the width of your rectangle:”
Input width.
Display area.
Program of Area of Rectangle in Javascript
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
<html> <head> <title>Area of Rectangle</title> </head> <style> h3 { font-family:Bodoni MT; }; </style> <body> <script> var L = parseInt(prompt("Enter the length of your Rectangle : ")); var W = parseInt(prompt("Enter the width of your Rectangle : ")); var area = (L * W); document.write("<h3> Area of the Rectangle</h3>"); document.write(" The Length of the Rectangle is " + L + ".</font><br>"); document.write(" The Width of the Rectangle is " + W + ".</font><br><br>"); document.write(" The Area of the Rectangle is " + area +".</font>"); </script> </body> </html> |
Output
Area of the Rectangle
The length of the rectangle is 45.
The width of the rectangle is 45.
The area of the rectangle is 2025.
Even Odd Program in Javascript
Algorithm of Even-Odd Program in Javascript
1) start
2 ) create function odd-even
3) declare variable n;
4) if the module of variable n with 2 is 0 then show even number
5) else show an odd number
Pseudocode of Even-Odd Program in Javascript
Checking even or odd number
1) Begin
2) declare variable n
3) if n%3=0 print even
4) else print odd
5) end
Program of Even-Odd Program in Javascript
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
<!doctype html> <html> <head> <script> function odd_even(){ var n; n=Number(document.getElementById("no_input").value); if(n%2==0) { document.write("Even Number"); } else { document.write("Odd Number"); } } </script> </head> <body> Enter Any Number:<input id="no_input"><br /> <button onclick="odd_even()">Click me</button> </body> </html> |
The minimum and maximum value in javascript
Flowchart of the minimum and maximum value
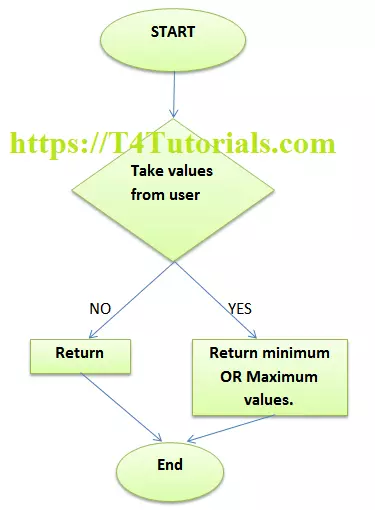
Algorithm of the minimum and maximum value in javascript
- Declare an array.
- Initialize array.
- Compare the first index value with all other values of an array.
- Print maximum OR minimum value.
Pseudo code for maximum value
- Max = 0;
- Declare and initialize array.
- Max = a[0];
- For loop to array size;
- if ( a [ I ] > max ) ;
- Print value max variable;
Pseudo code minimum value
- Min = 0;
- Declare and initialize array.
- Min = a [ 0 ];
- For loop to array size.
- if ( a [ I ] < min)
- Print value max OR min variable.
Program of the minimum and maximum value in javascript
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
< ! DOCTYPE html > < html > < head > < title >find maximum and minimum values in array in js < / title > < script type = " text / javascript " > function minValue ( ) { var a =document.getElementById( " val ") . value ; var aa = parseInt( a ) ; document.write ( " Minimum value is : " + Math.min.apply ( aa ) ) ; } function maxValue ( ) { var a =document.getElementById ( " val " ).value ; var aa = parseInt ( a ) ; document.write ( " Maximum value is : " + Math.max.apply ( aa ) ); } < / script > < / head > < body > < form name = " form233 "> Enter values : < input id = " val " type = " text " name = " value " > < input type = " button " name = " min " onclick = "minValue ( ) " value = " Click for Minimum value " > < input type = " button " name = " mix " onclick = "maxValue ( ) " value = " Click for Maximum value " > < / f orm > < / body > < / html > |
Concatenation of Strings in Javascript
Algorithm for Concatenation of String
First, take some string and store this string into any variable.
Take another string and store it in another variable.
Concatenate variables.
PseudoCode for Concatenation of String
- String one = any string
- String two = any string
- String three = string one. Cancat (string two);
- Display string three
Program of Concatenation of Strings in Javascript
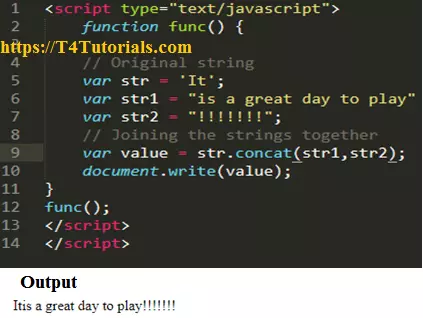
Reverse the Number in Javascript
Pseudocode to Reverse the Number in Javascript
- Create html tag
- Make <button onclick revers function ()
- Give id = to “rev”</p>
- Start <script>
- Array list[1,2,4,5,7,90,34,];
- By .innerHTML equal = num;
- Get id , to “rev”
- Make fun backward();{
- Compare No:1.backward();
- Get value getelementbyid(“rev”)
- }
- </script>
- Close </body>
- </html>
Algorithm of Reverse the Number in Javascript
Create html tag
Make <button onclick revers function ()
Give id = to “rev”</p>
Start <script>
Array list[1,2,4,5,7,90,34,];
By .innerHTML equal = num;
Get id , to “rev”
Make fun backward();{
Compare No:1.backward();
Get value getelementbyid(“rev”)
}
</script>
Close </body>
</html>
Program to Reverse the Number in Javascript
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<html> <body> <button onclick="reverse()">Click Here to reverse the number</button> <p id="rev"></p> <script> var num = ["9", "5", "2", "3"]; document.getElementById("rev").innerHTML = num; function reverse() { num.reverse(); document.getElementById("rev").innerHTML = num; } </script> </body> </html> |
Output
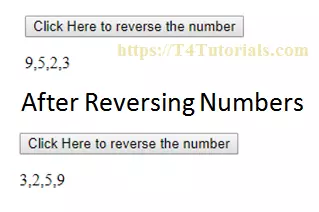
Javascript Program for converting hours into minutes and seconds
Algorithm for converting hours into minutes and seconds
1-start
2-get input from the user,
3-for find minutes n*60.
4-For find seconds n*3600.
5-creat function.
6- call function.
7- stop.
Javascript Program for converting hours into minutes and seconds
Swapping Values Program in Javascript
Algorithm of Swapping Values Program
Algorithm:
Step1: Start.
Step:2 Read values a and b.
Step3: a=a+b
b=a-b
a=a-b
Step4: Print a and b
Step5: Stop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
package com.java2novice.algos; public class MySwapingTwoNumbers { public static void main(String a[]){ int a = 10; int b = 20; System.out.println("Before swaping:"); System.out.println("a value: "+a); System.out.println("b value: "+b); a = a+b; b=a-b; a=a-b; System.out.println("After swaping :"); System.out.println("a value: "+a); System.out.println("b value: "+b); } } |