How to Inherit the Classes in JavaScript?
Inheritance in JavaScript describes the notion that one object’s methods/properties are available to be used via another object. … But with the delegation, those changes are then available, which has a term in the inheritance world: retroactive inheritance.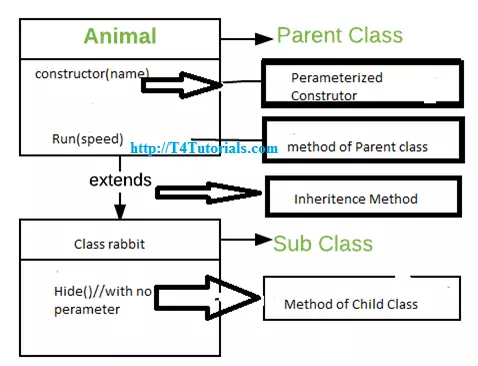
Algorithm of Classes Inheritance in JavaScript
- Open Notepad/notepad++/Dreamweaver
- Create new
- First include <html> tag ,<body> tag
- Declare a class with the name of Animal with class keyword
- Create constructor with constructor keyword and pass parameters of name
- Assign values in name and speed variables through constructor
- Create a method for speed with the name of Run and pass speed as a perimeter
- Increase the value of speed initially by 1.
- Print the value of speed with an animal name
- Create new class rabbit with keyword Class
- Inherit this class with parent class Animal by using the keyword extends
- Create a new method with the name of hiding for giving value in the name.
- Create an object of rabbit class with the name of Rabbit
- At the time of the creation of an object called the parameterized constructor to give value in the name “white”.
- Call the method of Run and Hide with this object.
Pseudocode of Classes Inheritance in JavaScript
<Html> <Body> Create Class Animal Create parameterized constructor pass parameter (name) Give value speed = 0; this.name = name run (speed) //create method this. speed += speed;// increase value of speed with 1 print this.name} runs with speed this.speed create a new method speed() this.speed = 0; print this.name stopped class Rabbit extends Animal hide() { PRINT this.name hides Create OBJECT rabbit = new Rabbit White Rabbit rabbit.run 5. Rabbit. Hide(); // White Rabbit hides!
Program of Classes Inheritance in JavaScript
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
this.speed = 0; this.name = name; } run(speed) { this.speed += speed; alert(`${this.name} runs with speed ${this.speed}.`); } stop() { this.speed = 0; alert(`${this.name} stopped.`); } } // Inherit from Animal class Rabbit extends Animal { hide() { alert(`${this.name} hides!`); } } let rabbit = new Rabbit("White Rabbit"); rabbit.run(5); // White Rabbit runs with speed 5. rabbit.hide(); // White Rabbit hides! </script> </body> </html> |
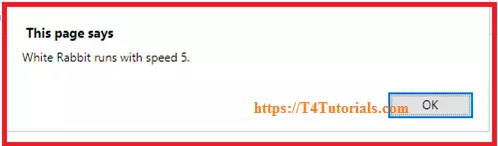