Factorial Program in C, C++ (C Plus Plus, CPP) with flow chart
In this tutorial, we will learn about the followings;- Flowchart of the factorial program
- C++ program for factorial program
- C program for factorial program
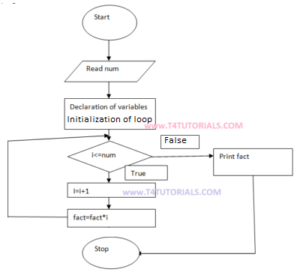
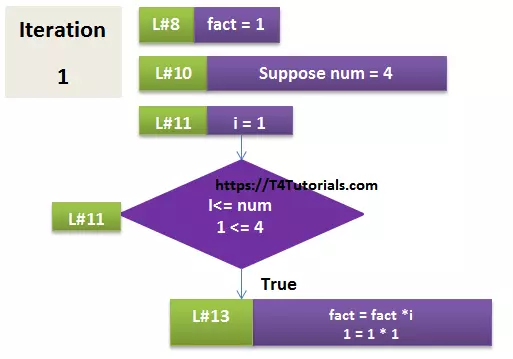
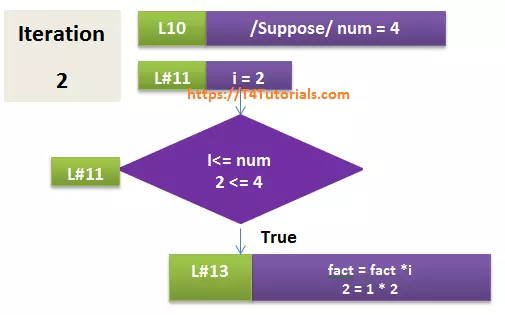
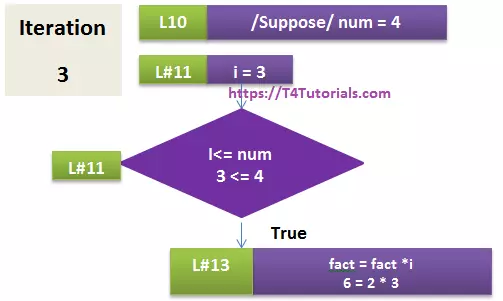
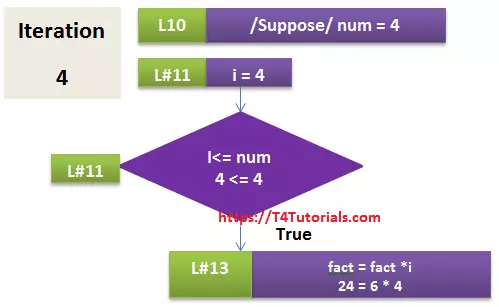
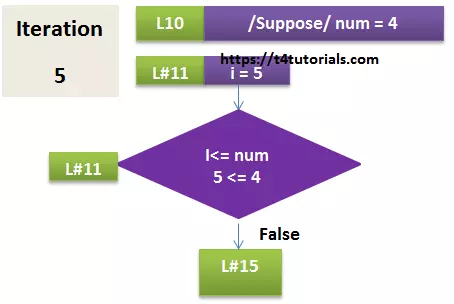
C++ program for factorial program
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
#include<iostream> #include<conio.h> using namespace std; int main() { int i; int num; float fact=1; cout<<"Please enter a number: "; cin>>num; for(i=1;i<=num;i++) { fact=fact*i; } cout<<"Factorial of entered number is: "<<fact; getch(); } |

SFT (Shamil’s Flow Table )
Are you interested to Read about SFT(Shamil’s Flow Table)?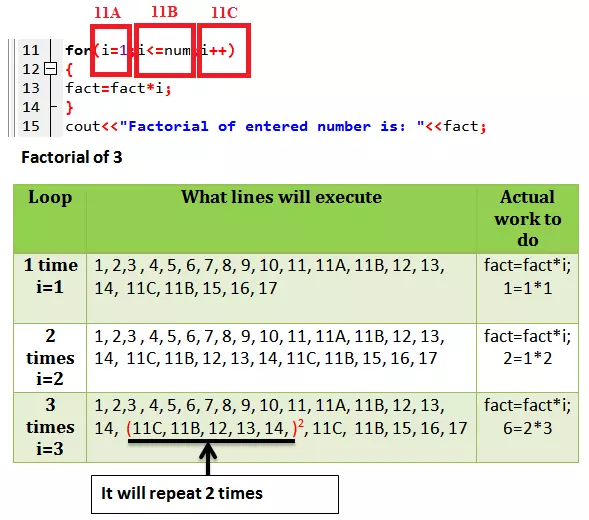
Factorial Program in C++ using while loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
#include<iostream> #include<conio.h> using namespace std; int main() { int n,Factorial=1,loop=1; cout<<"\n Enter The Number:"; cin>>n; //LOOP TO CALCULATE THE FACTORIAL OF A NUMBER while(loop<=n) { Factorial=Factorial*loop; loop++; } cout<<"\n The Factorial of "<<n<<" is "<<Factorial; getch(); } |
Factorial Program in C++ using do while loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
#include<iostream> #include<conio.h> using namespace std; int main() { int loop=1,Number,factorial=1; cout<<" Enter Any Number: "; cin>>Number; do { factorial=factorial*loop; loop++; }while(loop<=Number); cout<<"\n factorialorial of given Number is: "<<factorial; getch(); } |
C++ Factorial Program using Recursion
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
#include<iostream> using namespace std; int factorial(int MyNumber); int main() { int MyNumber; cout << "Enter a positive integer: "; cin >> MyNumber; cout << "Factorial of " << MyNumber << " = " << factorial(MyNumber); return 0; } int factorial(int MyNumber) { if(MyNumber > 1) return MyNumber * factorial(MyNumber - 1); else return 1; } |
Factorial Program in C++ using user define function
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#include<iostream> #include<conio.h> using namespace std; //Function long T4Tutorials(int); int main() { // Variable Declaration int LoopCounter, n; // Get Input Value cout << "Please Enter the Number :"; cin>>n; cout << n << " T4Tutorials Value Is " << T4Tutorials(n); // Wait For Output Screen getch(); return 0; } long T4Tutorials(int n) { int LoopCounter; long fact = 1; //for Loop Block for (int LoopCounter = 1; LoopCounter <= n; LoopCounter++) { fact = fact * LoopCounter; } return fact; } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
#include <stdio.h> #include<conio.h> int main() { int i; int num; unsigned long long fact = 1; printf("Please Enter a number: "); scanf("%d",&num); for(i=1; i<=num;i++) { fact *= i; } printf("Factorial of Entered number is %llu", fact); getche(); } |

More Practice on Factorial problem in C++
- Factorial Program in C++
- factorial using single inheritance
- Factorial Program in C++ using Class Objects
- factorial using Multiple inheritances
C++ program for factorial using Constructor DestructorFactorial Of A Number By Using The Recursion - Factorial Program with structures and pointers C++
- Factorial Program with Nested Structure C++
- factorial of a no. by defining the member functions outside the class