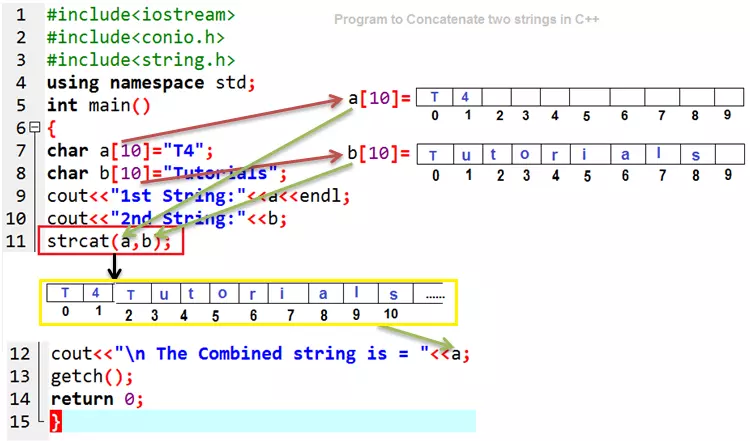
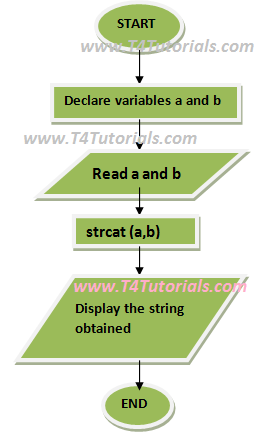
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
#include<iostream> #include<conio.h> #include<string.h> using namespace std; int main() { char a[10]="T4"; char b[10]="Tutorials"; cout<<"1st String:"<<a<<endl; cout<<"2nd String:"<<b; strcat(a,b); cout<<"\n The Combined string is = "<<a; getch(); return 0; } |
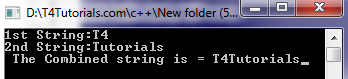
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
#include <stdio.h> #include <string.h> int main() { char a[100],b[100]; printf("enter 1st string:"); gets(a); printf("\nenter 2nd string:"); gets(b); strcat(a,b); printf("string obtained on cantenation is %s=\n",a); return 0; } |
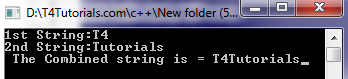
Program of Concatenation of Strings in C++
“Program of Concatenation of Strings in C++” is the today topic of discussion in this tutorial.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
#include<iostream> #include<string.h> using namespace std; int main() { int i; char a[10]="T4",b[10]="Tutorials"; for(i=1;i<=2;i++) { cout<<"\n\n1st string = "<<a<<endl; cout<<"2nd string = "<<b<<endl; strcat(a,b); cout<<"The combined string is = "<<a; } } |
Program of Concatenation of Strings in C++ Using Do While Loop
“Program of Concatenation of Strings in C++ Using Do While Loop” is the today topic of discussion in this tutorial.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
#include<iostream> #include<string.h> using namespace std; int main() { int i=1; char a[10]="T4",b[10]="Tutorials"; do { cout<<"\n\n1st string = "<<a<<endl; cout<<"2nd string = "<<b<<endl; strcat(a,b); cout<<"The combined string is = "<<a; i++; } while(i<=2); } |
Program of Concatenation of Strings in C++ Using Switch Statement
“Program of Concatenation of Strings in C++ Using Switch Statement” is the today topic of discussion in this tutorial. char control(char op) is the user define a function in this example.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
#include<iostream> #include<string.h> using namespace std; int main() { char op; cout<<"enter op Y or N"<<endl; cin>>op; switch(op) { case 'Y': { char a[10]="T4",b[10]="Tutorials"; cout<<"1st string = "<<a<<endl; cout<<"2nd string = "<<b<<endl; strcat(a,b); cout<<"The combined string is = "<<a; break; } case 'N': { cout<<"you have entered N means NO"<<endl; break; } default: cout<<"invalid op"; } } |
Program of Concatenation of Strings in C++ Using User Define Functions
“Program of Concatenation of Strings in C++ Using User Define Functions” is the today topic of discussion in this tutorial.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
#include<iostream> #include<string.h> using namespace std; char control(char); int main() { char c,op; c=control(op); } char control(char op) { cout<<"enter op"<<endl; cin>>op; if(op=='Y') { char a[10]="T4",b[10]="Tutorials"; cout<<"1st string = "<<a<<endl; cout<<"2nd string = "<<b<<endl; strcat(a,b); cout<<"The combined string is = "<<a; } else cout<<"invaid op"; return op; } |
Video Lecture