How to check if a sentence is a palindrome in C++?
We need to read from left and right, if the word is the same, whether we read it from left or we read it from right, then its called palindrome.
Example of palindrome
For example, “t4tutorials” is not a palindrome but “t4t” is a palindrome.
Write a C++ program to show whether a number is a palindrome or not using a do-while loop?
Flowchart of Palindrome Program using do-while loop
Code of Palindrome Program using do-while loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
#include<iostream> using namespace std; int main() { int num,r,sum=0,t; cout<<"enter number"<<endl; cin>>num; t=num; do { r=num%10; sum=sum*10+r; num=num/10; } while(num!=0); if(t==sum) { cout<<"The number is a palindrome:"; } else cout<<"Number is not palidrome:"; } |
Output
enter number
575
The number is a palindrome:
Suppose the input value is: 121
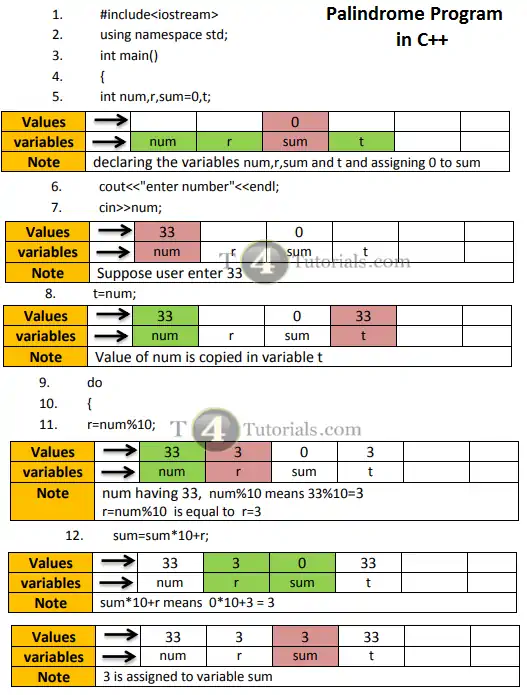
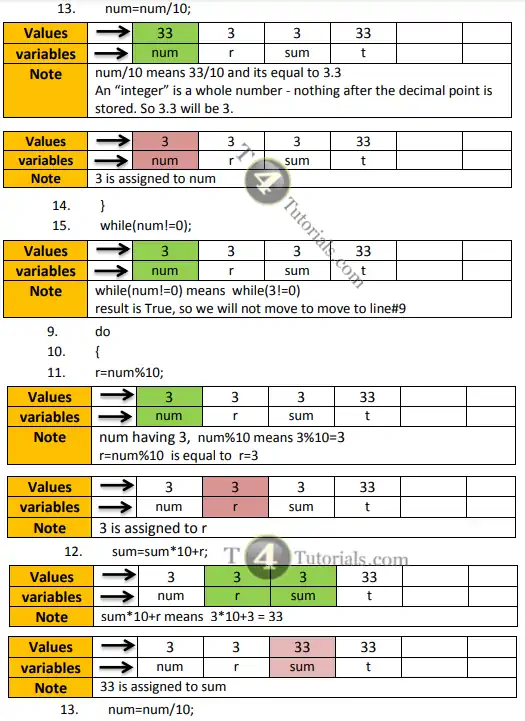
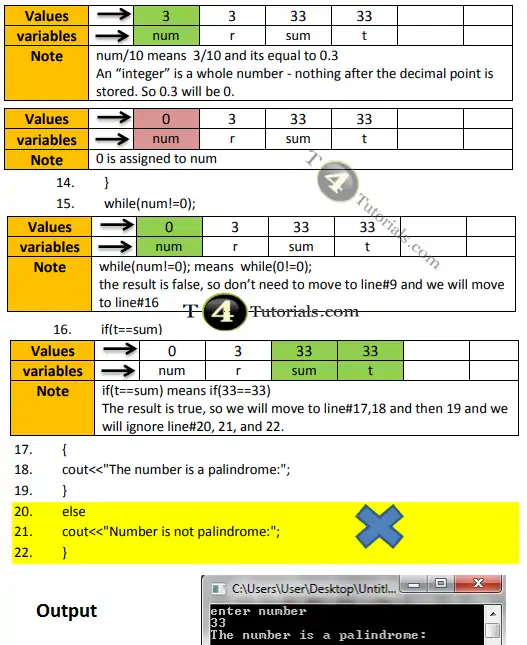
Exercise
Find the possible mistakes in the following Shamil’s Flow Table of the program to show whether a number is a palindrome or not using a do-while loop?
Loop execution | Condition
Num !=0 |
What line will execute? | Operations performed |
1st time | True | 1,2,3,4,5,6,7,8,9,10,11,12,13,14,15 | r=12%10=2
sum=0*10+2=2 num=121/10=12 |
2nd time | False | 1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,9,10,
11,12,13,14,15, 16,17,18,19,22 |
r=1%10=1 sum=12*10+1=121 num=12/10=1 |
2nd time | false | 1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,9,10,
11,12,13,14,15, 16,20,21,22 |
|
Flowchart of Palindrome Program using for loop
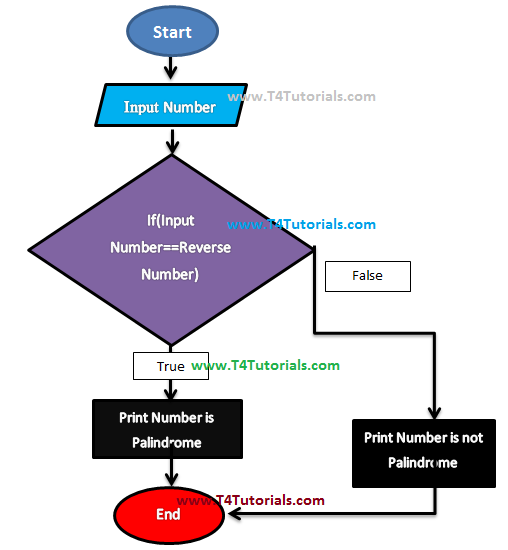
Palindrome Program using for loop for checking the numbers as a palindrome
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
#include <iostream> #include <conio.h> using namespace std; int main() { int Input_Num; int Orignal_Num; int Reverse_Num; int Remainder=0; cout<<"Enter an Integer number:\n"; cin>>Input_Num; Orignal_Num=Input_Num; int lengthCount = 0; for(; Input_Num != 0;) { Reverse_Num=Input_Num%10; Input_Num /= 10; Remainder=Remainder*10+Reverse_Num; Reverse_Num=Remainder; } if(Orignal_Num==Reverse_Num) cout<<"Number You Entered Is Palindrome"; else cout<<"Number Is Not Palindrome!!!"; getch(); } |
Output
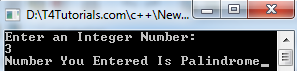
Palindrome Program using for loop for checking the strings as a palindrome
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
#include<iostream> using namespace std; int main() { int i,j,Number_Length,T4Tutorials_Flag=1; char a[20]; cout<<"Enter a string:"; cin>>a; for(Number_Length=0;a[Number_Length]!='\0';++Number_Length); for(i=0,j=Number_Length-1;i<Number_Length/2;++i,--j) { if(a[j]!=a[i]) T4Tutorials_Flag=0; } if(T4Tutorials_Flag==1) cout<<"\nThe string is Palindrome"; else cout<<"\nThe string is not Palindrome"; return 0; } |
Palindrome Program in C++ using user define function
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
#include<stdio.h> int checkPalindrome(int Num) { int Temporary, remainder, T4Tutorials=0; Temporary = Num; while( Num!=0 ) { remainder = Num % 10; T4Tutorials = T4Tutorials*10 + remainder; Num /= 10; } if ( T4Tutorials == Temporary ) return 0; else return 1; } int main() { int Num; printf("Please! Enter the Num: "); scanf("%d", &Num); if(checkPalindrome(Num) == 0) printf("%d is a palindrome Num.\n",Num); else printf("%d is not a palindrome Num.\n",Num); return 0; } |
Output
Please! Enter the Num: 4
4 is a palindrome Num.
——————————–
Palindrome recursion C++
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
#include <iostream> using namespace std; //Writing a Recursive function to check if string is a palindrome or not bool isPalindrome(string str, int X, int Y) { // base case if (X >= Y) return true; // return false if mismatch happens if (str[X] != str[Y]) return false; // move to next the pair return isPalindrome(str, X + 1, Y - 1); } int main() { string str = "T4Tutorials"; int len = str.length(); if (isPalindrome(str, 0, len - 1)) cout << "its Palindrome"; else cout << "sorry, its Not Palindrome"; return 0; } |
Palindrome Program in C
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
#include <conio.h> int main() { int Input_Num; int Orignal_Num; int Reverse_Num; int Remainder=0; printf("Enter an Integer Number:\n"); scanf("%d", &Input_Num); Orignal_Num=Input_Num; int lengthCount = 0; for(; Input_Num != 0;) { Reverse_Num=Input_Num%10; Input_Num /= 10; Remainder=Remainder*10+Reverse_Num; Reverse_Num=Remainder; } if(Orignal_Num==Reverse_Num) printf("Number You Entered Is Palindrome"); else printf("Number Is Not Palindrome!!!"); getch(); } |
Output
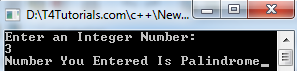