Difference between Early binding and late binging
Early binding | late binging |
Order of Execution: 1st(Early binding) then 2nd (late binging) | |
static binging | Dynamic Binding |
Compile-time | Run time polymorphism |
Matching of a function call with function definition is performed at compiler time. | Matching of a function call with function definition is performed at run time. |
Full information about function calling and matching with function definition is visible to the compiler during compile time | Full information about function calling and matching with function definition is not visible to the compiler during compile time but it’s visible during run time. |
More efficient: because the compiler already has knowledge that what code will execute | More flexible: |
Fast execution | Slow execution |
Examples: Function overloading and operator overloading | Examples: Virtual functions |
Example of Early binding in C++
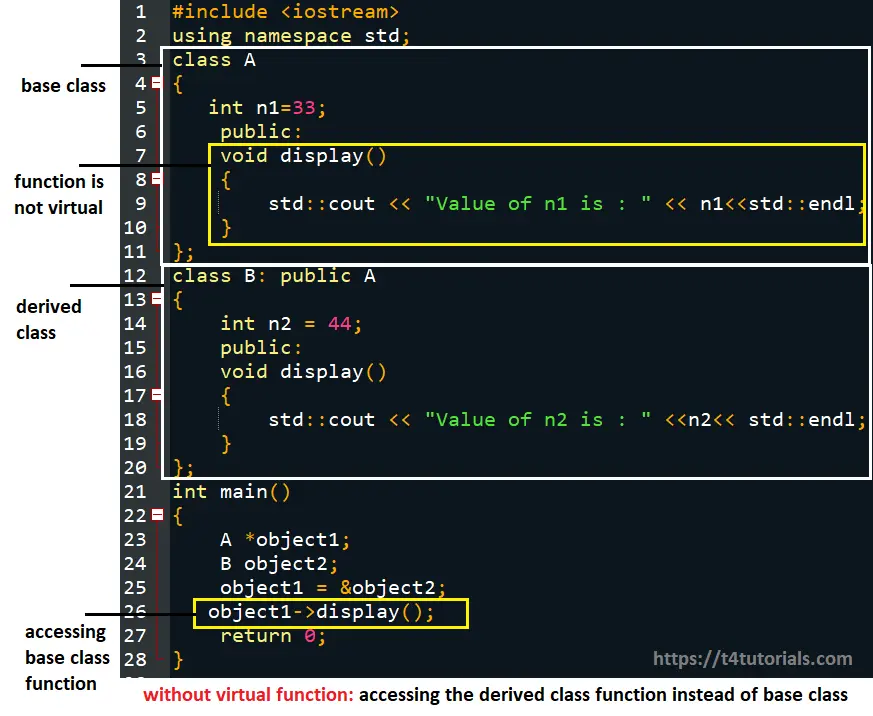
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
#include <iostream> using namespace std; class A { int n1=33; public: void display() { std::cout << "Value of n1 is : " << n1<<std::endl; } }; class B: public A { int n2 = 44; public: void display() { std::cout << "Value of n2 is : " <<n2<< std::endl; } }; int main() { A *object1; B object2; object1 = &object2; object1->display(); return 0; } |
Example of Late binding in C++
Late binding can be implemented by making a function as a virtual function.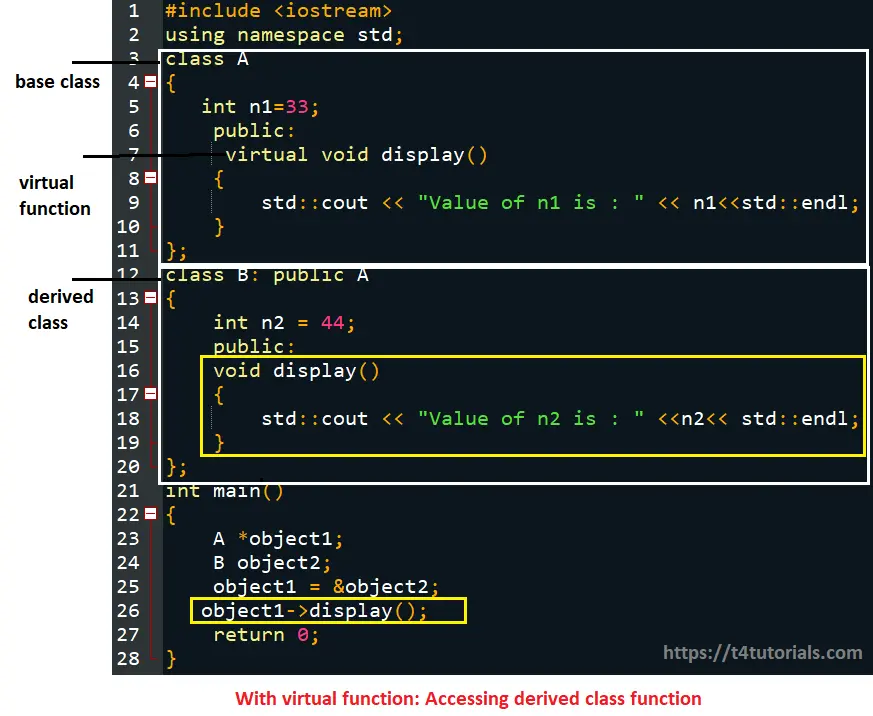
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
#include <iostream> using namespace std; class A { int n1=33; public: virtual void display() { std::cout << "Value of n1 is : " << n1<<std::endl; } }; class B: public A { int n2 = 44; public: void display() { std::cout << "Value of n2 is : " <<n2<< std::endl; } }; int main() { A *object1; B object2; object1 = &object2; object1->display(); return 0; } |