Write a program that lets the user enter the total rainfall for each of 12 months.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
#include <iostream> #include <string> #include <iomanip> using namespace std; int main () { const int ALLMONTHS = 12; string name[ALLMONTHS]= {"January","February","March","April","May","June","July","August","September","October","November","December"}; int LoopVariable= 0; double rain[ALLMONTHS]; double avg; double year=0; double maximum; string highMonth; double minimum; string lowMonth; for(LoopVariable = 0; LoopVariable < ALLMONTHS; LoopVariable++) // ask user to enter amount of rainfall for each month { cout <<"How many inches of rain does "<< name[LoopVariable]; cout<< " have? \n"; cin >> rain[LoopVariable]; while (rain[LoopVariable] < 0) { cout << "Please enter a number greater than 0."<< endl; cin >> rain[LoopVariable]; } } for(int LoopVariable=0; LoopVariable<ALLMONTHS; LoopVariable++) // totals up all the rainfall year += rain[LoopVariable]; avg = year / ALLMONTHS; for( int LoopVariable = 0; LoopVariable < ALLMONTHS; LoopVariable++) //spits out each month with its amount of rain { cout << name[LoopVariable]; cout<< " has "; cout<< rain[LoopVariable] << " inches of rainfall.\n"; } maximum = rain[0]; // finds month with the maximum amount of rain for (LoopVariable = 1 ;LoopVariable < ALLMONTHS; LoopVariable++) { if (rain[LoopVariable] > maximum) { highMonth = name[LoopVariable]; maximum = rain[LoopVariable]; } } minimum = rain[0]; // finds month with the least amount of rain for (LoopVariable = 1 ;LoopVariable < ALLMONTHS; LoopVariable++) { if (rain[LoopVariable] < minimum) { lowMonth = name[LoopVariable]; minimum = rain[LoopVariable]; } } cout << endl; cout << setprecision(2) << fixed; cout <<"Total Rainfall in the year is total" <<year << " inches" << endl; cout <<"The average monthly rainfall is total" << avg << " inches"<< endl; cout <<"The month with the maximum amount of rainfall is total " << highMonth << " with " << maximum<< " inches."<< endl; cout <<"The month with the minimum amount of rainfall is total" << lowMonth << " with " << minimum<< " inches."<< endl; return 0; } |
Output
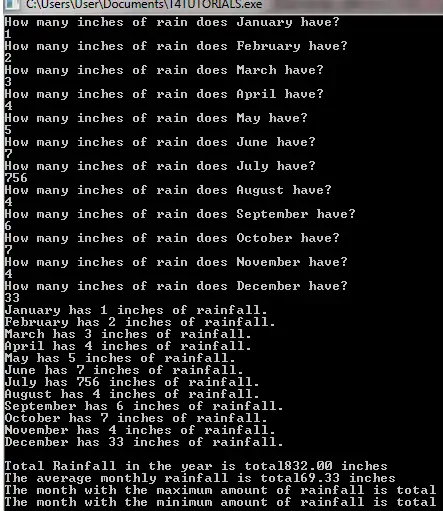