Linked List Insert Traverse Delete Implementation & Operations in Data Structures (C++)
In this tutorial, we will learn about Linked List Insert Traverse Delete Implementation & Operations in Data Structures (C++).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 |
//C++ Code Snippet - Linked List Implementation using C++ Program #include <iostream> using namespace std; //Declaration of Node struct Node{ int number; Node *next; }; //Declaration of Head node struct Node *head=NULL; //Inserting node at start void node_insertion(int a) { struct Node *newNode=new Node; newNode->number=a; newNode->next=head; head=newNode; } //Traversing/displaying entered nodes void show() { if(head==NULL) { cout<<" Linked List is empty!"<<endl; return; } struct Node *temp=head; while(temp!=NULL) { cout<<temp->number<<" "; temp=temp->next; } cout<<endl; } //Deleting node from start void del_Item() { if(head==NULL){ cout<<"Linked List is empty!"<<endl; return; } cout<<head->number<<" is removed."<<endl; head=head->next; } int main() { show(); node_insertion(15); node_insertion(30); node_insertion(45); node_insertion(60); node_insertion(75); show(); del_Item(); del_Item(); del_Item(); del_Item(); del_Item(); del_Item(); show(); return 0; } |
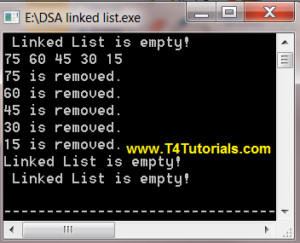