Stack Push Pop Traverse Implementation and Operations in Data Structures (C plus plus)
In this tutorial, we will learn Stack Push Pop Traverse Implementation and Operations in Data Structures (C plus plus).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 |
/* Implementation of Stack through Linked List */ #include<iostream> #include<cstdlib> using namespace std; /* Declaring the node */ struct node { int info; struct node *link; }*top; /* Declaring the class */ class stack_vals { public: node *push(node *, int); node *pop(node *); void traverse(node *); stack_vals() { top = NULL; } }; int main() { int val_selection, object; stack_vals sl; while (1) { cout<<"\n----------"<<endl; cout<<"Stack Operations:"<<endl; cout<<"\n----------"<<endl; cout<<"1. Push Element"<<endl; cout<<"2. Pop Element"<<endl; cout<<"3. Traverse"<<endl; cout<<"4. Quit"<<endl; cout<<"Please Enter your choice:"; cin>>val_selection; switch(val_selection) { case 1: cout<<"Please enter the desired value: "; cin>>object; top = sl.push(top, object); break; case 2: top = sl.pop(top); break; case 3: sl.traverse(top); break; case 4: exit(1); break
; default: cout<<"Invalid input"<<endl; } } return 0; } /* Push Element */ node *stack_vals::push(node *top, int object) { node *temporary; temporary = new (struct node); temporary->info = object; temporary->link = top; top = temporary; return top; } /* Pop Element */ node *stack_vals::pop(node *top) { node *temporary; if (top == NULL) cout<<"Empty Stack"<<endl; else { temporary = top; cout<<"Element Popped Successfully: "<<temporary->info<<endl; top = top->link; delete(temporary); } return top; } /* * Traversing */ void stack_vals::traverse(node *top) { node *temp1; temp1 = top; if (top == NULL) cout<<"Empty Stack"<<endl
; else { cout<<"Elements in stack are following:"<<endl; while (temp1 != NULL) { cout<<temp1->info<<endl; temp1 = temp1->link; } } } |
Output:
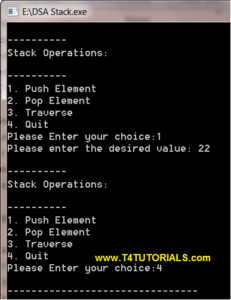