Program to Implement Priority Queue in Data Structures (C plus plus)
In this tutorial, we will learn about the Program to Implement Priority Queue in Data Structures (C plus plus).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 |
/*C++ Program to Implement Priority Queue*/ #include <iostream> #include <cstdlib> using namespace std; /*Declaring the node*/ struct node { int prior; int data; struct node *link; }; class priority_queue { private: node *front; public: priority_queue() { front = NULL; } void insertion(int item, int prior) { node *tmp, *q; tmp = new node; tmp->data = item; tmp->prior = prior; if (front == NULL || prior < front->prior) { tmp->link = front; front = tmp; } else { q = front; while (q->link != NULL && q->link->prior <= prior) q=q->link; tmp->link = q->link; q->link = tmp; } } void deletion() { node *tmp; if(front == NULL) cout<<"Queue Underflowed "<<endl; else { tmp = front; cout<<"Deleted value is: "<<tmp->data<<endl; front = front->link; free(tmp); } } void show() { node *ptr; ptr = front; if (front == NULL) cout<<"Empty Queue "<<endl; else { cout<<"Queue is: "<<endl; cout<<"Priority Value "<<endl; while(ptr != NULL) { cout<<ptr->prior<<" "<<ptr->data<<endl; ptr = ptr->link; } } } }; int main() { int ch, val, prior; priority_queue pq; do { cout<<"1. Insertion "<<endl; cout<<"2. Deletion "<<endl; cout<<"3. Display "<<endl; cout<<"4. Exit "<<endl; cout<<"Please enter your choice : "; cin>>ch; switch(ch) { case 1: cout<<"Please enter the value to be added in the queue : "; cin>>val; cout<<"Please enter its priority : "; cin>>prior; pq.insertion(val, prior); break; case 2: pq.deletion(); break; case 3: pq.show(); break; case 4: break; default : cout<<"Invalid entry"<<endl; } } while(ch != 4); return 0; } |
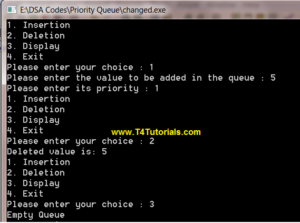