C++ Sort Array using Functions
“C++ Sort Array using Functions” is the topic of discussion in this tutorial.What is sorting in C++?
Sorting is the method of arranging the alphabets or numbers in ascending or descending or some another order. Ascending Alphabets order in C++: a, b, c, d, e, f, ……x, y, z Descending Alphabets order in C++: z, y, x, ……f, e, d, c, b, a Ascending Numbers order in C++: 0,1, 2, 3, 4, 5, 6, 7, 8, 9 Descending Alphabets order in C++: 9, 8, 7, 6, 5, 4, 3, 2, 1 Let’s begin with some examples of sorting the array.Example Program for Sorting the Array in C++
Following program covers the “Example Program for Sorting the Array in C++”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
#include<iostream> using namespace std; int main() { int array[100]; int size; int b; cout<<"enter number of values"<<endl; cin>>size; cout<<"enter values"<<endl; for(int i=0; i<size; i++) { cin>>array[i]; } for(int i=0; i<size; i++) { for(int j=i+1; j<size; j++) { if(array[i]>array[j]) { b=array[i]; array[i]=array[j]; array[j]=b; } } } cout<<endl<<"the sorted values are"<<endl; for(int i=0; i<size; i++) { cout<<array[i]; } } |
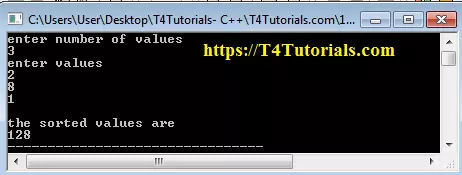
Program for Sorting the Array in C++ Using If Else Statement
Following program covers the “Program for Sorting the Array in C++ Using If Else Statement”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
#include<iostream> using namespace std; int main() { int array[100]; int size; int b; int t=1; int c; int cho; for(int i=0; i<t; i++) { system("cls"); cout<<"type 1 to run the program"<<endl; cin>>cho; if(cho!=1) { cout<<"you are now exiting..."<<endl; } else { cout<<"enter number of values"<<endl; cin>>size; cout<<"enter values"<<endl; for(int i=0; i<size; i++) { cin>>array[i]; } for(int i=0; i<size; i++) { for(int j=i+1; j<size; j++) { if(array[i]>array[j]) { b=array[i]; array[i]=array[j]; array[j]=b; } } } cout<<endl<<"the sorted values are"<<endl; for(int i=0; i<size; i++) { cout<<array[i]<<" "; } t=0; } } system("pause"); } |
Program for Sorting the Array in C++ Using While Loop
Following program covers the “Program for Sorting the Array in C++ Using While Loop”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
#include<iostream> using namespace std; int main() { int array[100]; int size; int b; cout<<"enter number of values"<<endl; cin>>size; cout<<"enter values"<<endl; int i=0; while(i<size) { cin>>array[i]; i++; } i=0; int j=1; while(i<size) { while(j<size) { if(array[i]>array[j]) { b=array[i]; array[i]=array[j]; array[j]=b; } j++; } i++; j=i+1; } cout<<endl<<"the sorted values are"<<endl; i=0; while(i<size) { cout<<array[i]<<" "; i++; } } |
Program for Sorting the Array in C++ Using Do While Loop
Following program covers the “Program for Sorting the Array in C++ Using Do While Loop”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
#include<iostream> using namespace std; int main() { int array[100]; int size; int b; cout<<"enter number of values"<<endl; cin>>size; cout<<"enter values"<<endl; int i=0; do { cin>>array[i]; i++; } while(i<size); i=0; int j=1; do { do { if(array[i]>array[j]) { b=array[i]; array[i]=array[j]; array[j]=b; } j++; } while(j<size); i++; j=i+1; } while(i<size); cout<<endl<<"the sorted values are"<<endl; i=0; do { cout<<array[i]<<" "; i++; } while(i<size); } |