Flowchart of the program to show the Sum of Array Elements
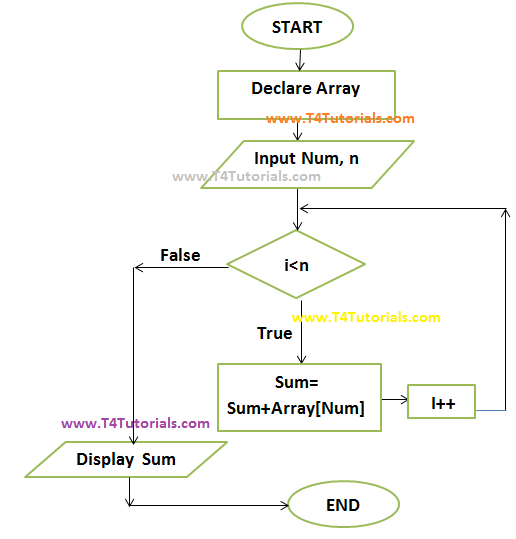
Program to show the Sum of Array Elements in C Plus Plus (CPP)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
#include<iostream> #include<conio.h> using namespace std; int main() { int Array[55]; int loop_1, loop_2, arary_size; int sum=0; cout<<"How many elements you want to enter: "; cin>>arary_size; cout<<"Enter the Elements:"; for(loop_1=0;loop_1<arary_size;loop_1++) { cin>>Array[loop_1]; } cout<<"Sum of Elements are: "; for(loop_2=0;loop_2<arary_size;loop_2++) { sum=sum+Array[loop_2]; } cout<<sum; getch(); } |
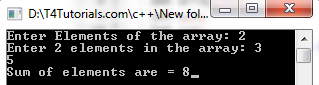
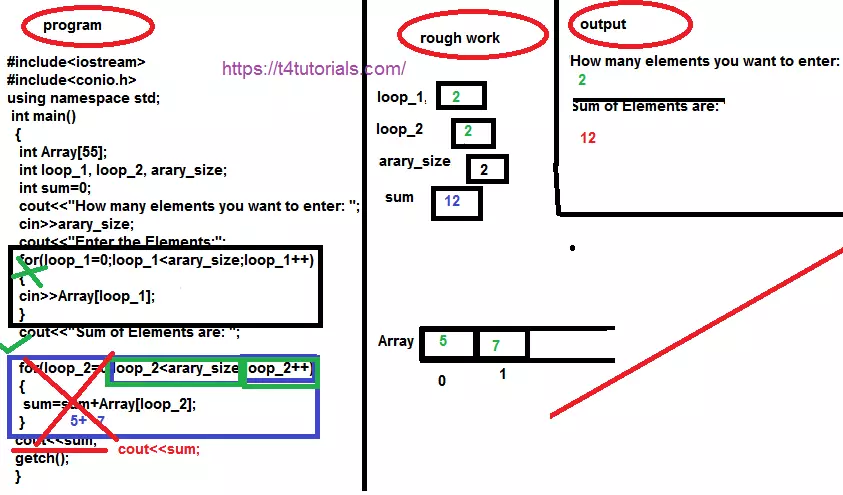
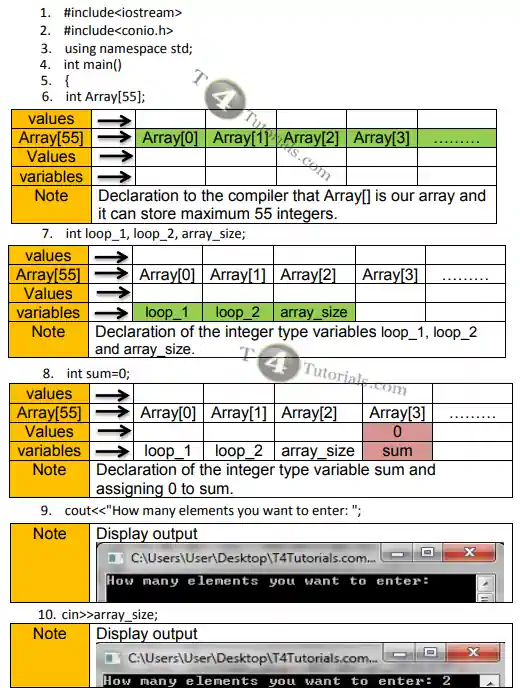
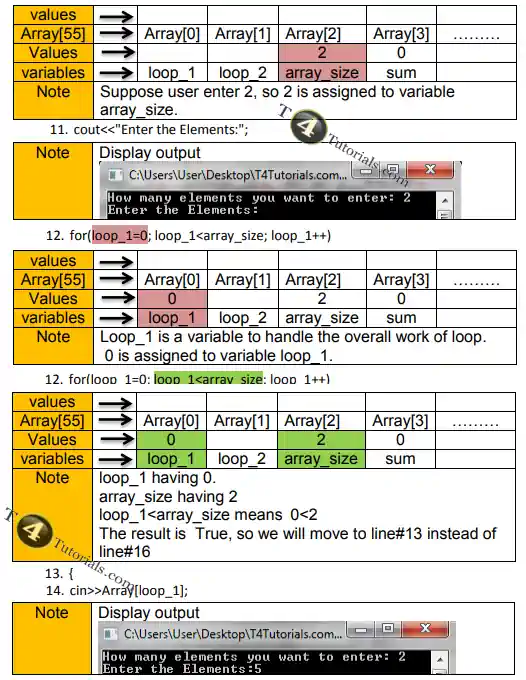
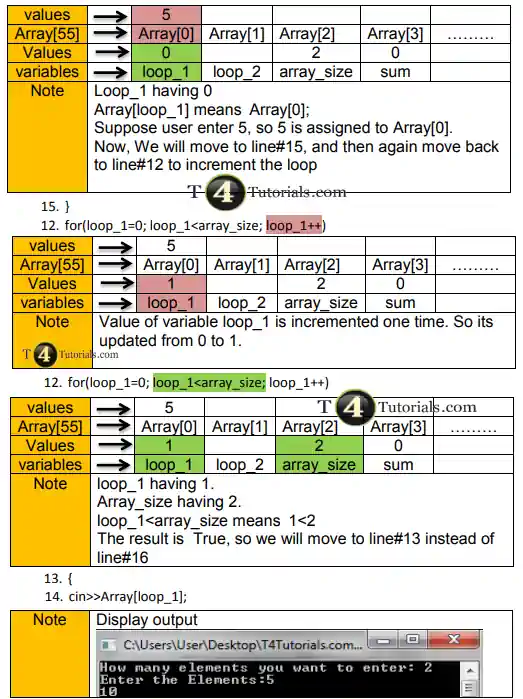
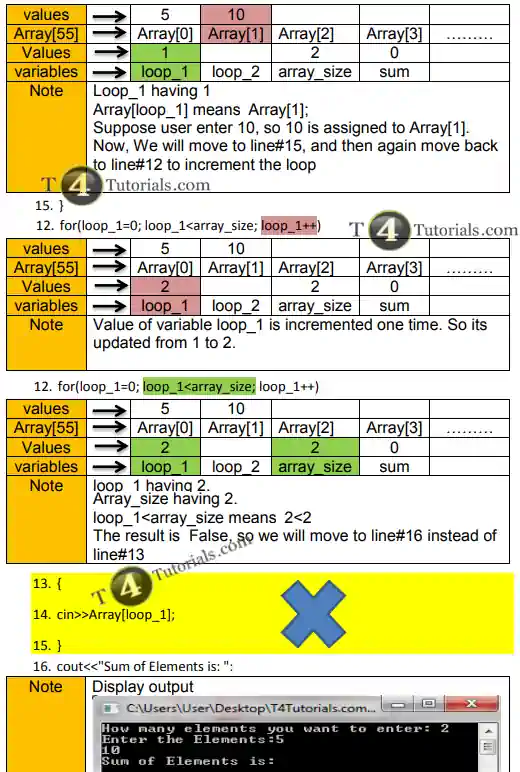
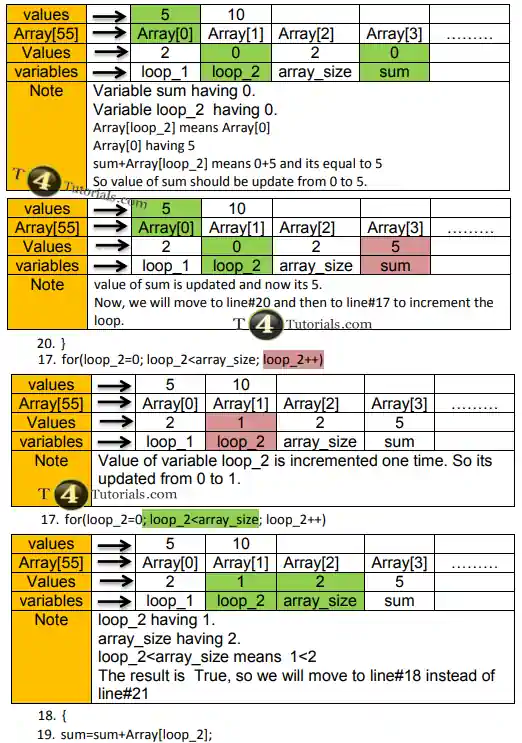
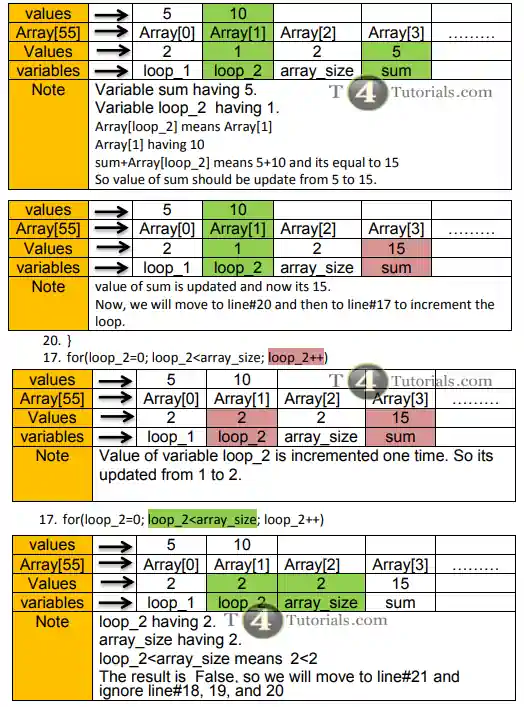
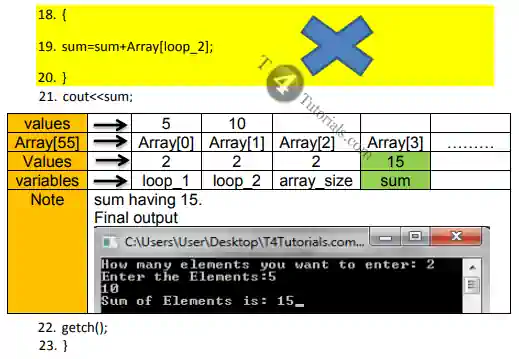
Program to show the Sum of Array Elements in C
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 |
#include <stdio.h> #include<conio.h> #define MAX_SIZE 20 void main() { int array[55]; int a,b; int sum; printf("Enter Elements of the array: "); scanf("%d", &b); printf("Enter %d elements in the array: ", b); for(a=0;a<b;a++) { scanf("%d", &array[a]); } for(a=0;a<b;a++) { sum = sum + array[a]; } printf("Sum of elements are = %d", sum); getch(); } |
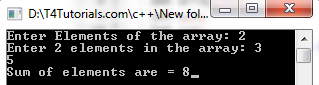
Sum of Array Elements in C++ using If Else Statement
Let’s see the program of “Sum of Array Elements in C++ using If Else Statement”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
#include<iostream> using namespace std; main() { int m, n, c, d; int first[10][10]; int second[10][10]; int sum[10][10]; int alert; cout<<"program is going to run; type 1 to agree.."<<endl; cin>>alert; if(alert!=1) { cout<<"you are going to exit"<<endl; } else { cout << "Enter the number of rows and columns of matrix "; cin >> m >> n; cout << "Enter the elements of first matrix\n"; for ( c = 0 ; c < m ; c++ ) for ( d = 0 ; d < n ; d++ ) cin >> first[c][d]; cout << "Enter the elements of second matrix\n"; for ( c = 0 ; c < m ;c++ ) for ( d = 0 ; d < n ; d++ ) cin >> second[c][d]; for ( c = 0 ; c < m ; c++ ) for ( d = 0 ; d < n ; d++ ) sum[c][d] = first[c][d] + second[c][d]; cout << "Sum of entered matrices:-\n"; for ( c = 0 ; c < m ; c++ ) { for ( d = 0 ; d < n ; d++ ) cout << sum[c][d] << "\t"; cout << endl; } } return 0; } |
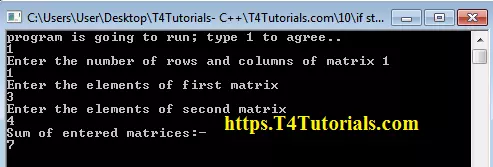
Sum of Array Elements in C++ using Nested For Loop
Let’s see the Program of “Sum of Array Elements in C++ using nested for loop”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
#include<iostream> using namespace std; main() { int m, n, c, d; int first[10][10]; int second[10][10]; int sum[10][10]; cout << "Enter the number of rows and columns of matrix "; cin >> m >> n; cout << "Enter the elements of first matrix\n"; for ( c = 0 ; c < m ; c++ ) for ( d = 0 ; d < n ; d++ ) cin >> first[c][d]; cout << "Enter the elements of second matrix\n"; for ( c = 0 ; c < m ;c++ ) for ( d = 0 ; d < n ; d++ ) cin >> second[c][d]; for ( c = 0 ; c < m ; c++ ) for ( d = 0 ; d < n ; d++ ) sum[c][d] = first[c][d] + second[c][d]; cout << "Sum of entered matrices:-\n"; for ( c = 0 ; c < m ; c++ ) { for ( d = 0 ; d < n ; d++ ) cout << sum[c][d] << "\t"; cout << endl; } return 0; } |
Sum of Array Elements in C++ using While Loop
Let’s see the Program of “Sum of Array Elements in C++ using while loop”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
#include<iostream> #include<iostream> using namespace std; main() { int m, n, c, d; int first[10][10]; int second[10][10]; int sum[10][10]; cout << "Enter the number of rows and columns of matrix "; cin >> m >> n; cout << "Enter the elements of first matrix\n"; while(c < m) { for ( d = 0 ; d < n ; d++ ) cin >> first[c][d]; c++; } cout << "Enter the elements of second matrix\n"; c=0; while (c < m) { for ( d = 0 ; d < n ; d++ ) cin >> second[c][d]; c++; } c=0; while (c < m) { for ( d = 0 ; d < n ; d++ ) sum[c][d] = first[c][d] + second[c][d]; c++; } cout << "Sum of entered matrices:-\n"; c=0; while (c < m) { for ( d = 0 ; d < n ; d++ ) cout << sum[c][d] << "\t"; c++; cout << endl; } return 0; } |
Sum of Array Elements in C++ using Do While Loop
Let’s see the Program of “Sum of Array Elements in C++ using do-while loop”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 |
#include<iostream> #include<iostream> using namespace std; main() { int m, n, c, d; int first[10][10]; int second[10][10]; int sum[10][10]; cout << "Enter the number of rows and columns of matrix "; cin >> m >> n; cout << "Enter the elements of first matrix\n"; do { for ( d = 0 ; d < n ; d++ ) cin >> first[c][d]; c++; } while(c < m); cout << "Enter the elements of second matrix\n"; c=0; do { for ( d = 0 ; d < n ; d++ ) cin >> second[c][d]; c++; } while (c < m); c=0; do { for ( d = 0 ; d < n ; d++ ) sum[c][d] = first[c][d] + second[c][d]; c++; } while (c < m); cout << "Sum of entered matrices:-\n"; c=0; do { for ( d = 0 ; d < n ; d++ ) cout << sum[c][d] << "\t"; c++; cout << endl; } while (c < m); return 0; } |
Sum of Array Elements in C++ using User Define Function in C++
Let’s see the Program of “Sum of Array Elements in C++ using the user-defined function”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
#include<iostream> using namespace std; int choose(int); int main() { int m, n, c, d; int first[10][10]; int second[10][10]; int sum[10][10]; char feedback; cout << "Enter the number of rows and columns of matrix "; cin >> m >> n; cout << "Enter the elements of first matrix\n"; for ( c = 0 ; c < m ; c++ ) for ( d = 0 ; d < n ; d++) cin >> first[c][d]; cout << "Enter the elements of second matrix\n"; for ( c = 0 ; c < m ;c++ ) for ( d = 0 ; d < n ; d++ ) cin >> second[c][d]; for ( c = 0 ; c < m ; c++ ) for ( d = 0 ; d < n ; d++ ) sum[c][d] = first[c][d] + second[c][d]; int value=choose(feedback); switch(value) { case 1: { cout << "Sum of entered matrices:-\n"; for ( c = 0 ; c < m ; c++ ) { for ( d = 0 ; d < n ; d++ ) cout << sum[c][d] << "\t"; cout << endl; } break; } default: { cout<<"program is going to exit"<<endl; } return 0; } } int choose(int val) { cout<<"please type '1' to display the sum, or hit any key to exit"<<endl; cin>>val; return val; } |
Video Lecture
Topic Covered the sum of elements in 1D(1 dimensional) array in c++. | sum of elements in an array C++ | How to sum all elements of one array in C++? | Adding elements of an array in C++? | simple array sum in c++ | sum of array elements using for loop in C++ | Step by step dry run of sum of array elements in C++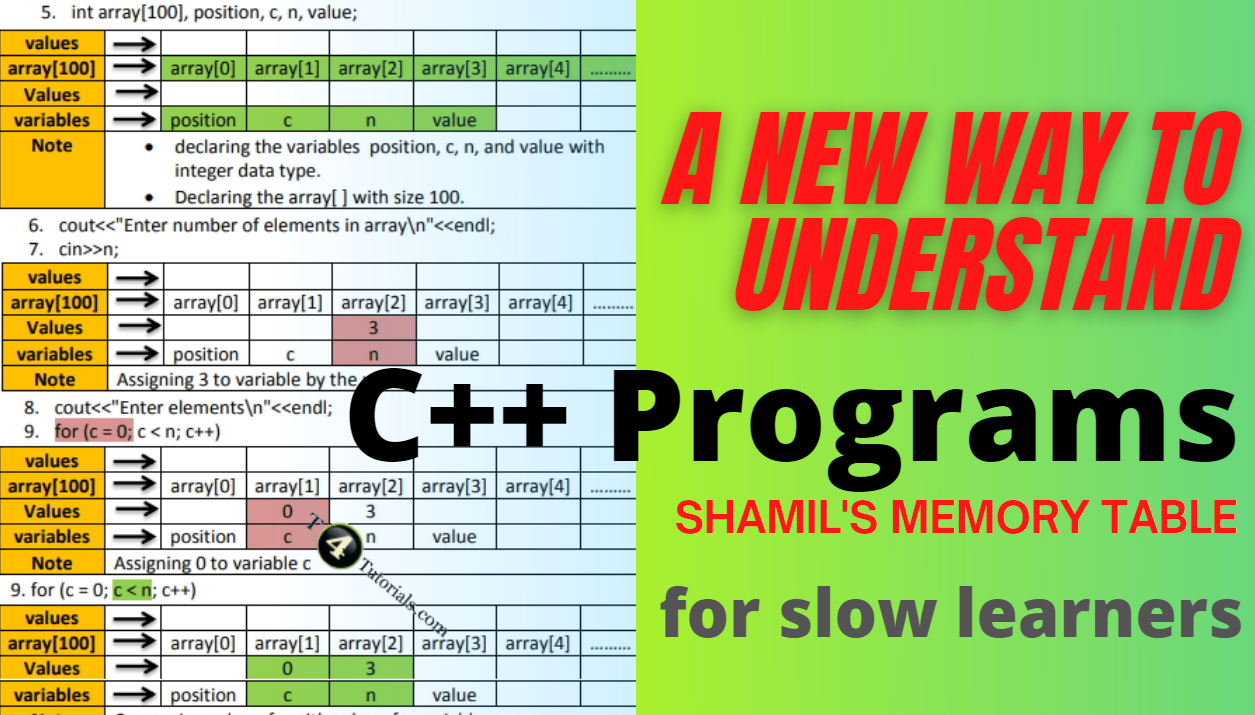