Program to evaluate an expression using stacks in Data Structures (C plus plus)
In this tutorial, we will learn about the Program to evaluate an expression using stacks in Data Structures (C plus plus).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 |
/*C++ Program to Evaluate an Expression using Stacks*/ #include <iostream> #include <string.h> using namespace std; struct node { int info; node *next; } *p = NULL, *top = NULL, *save = NULL, *ptr; void insert(int x) { p = new node; p->info = x; p->next = NULL; if (top == NULL) { top = p; } else { save = top; top = p; p->next = save; } } char del() { if (top == NULL) { cout<<"Underflowed "; } else { ptr = top; top = top->next; return(ptr->info); delete ptr; } } int main() { char z[30]; int x, y; cout<<"Please enter the balanced expression"<<endl; cin>>z; for (int u = 0; u < strlen(z); u++) { if (z[u] >= 48 && z[u] <= 57) insert(z[u]-'0'); else if (z[u] >= 42 && z[u] <= 47) { x=del(); y=del(); switch(z[u]) { case '+': insert(x+y); break; case '-': insert(x-y); break; case '*': insert(x*y); break; case '/': insert(x/y); break; } } } cout<<"The answer is "<<del()<<endl; } |
Output
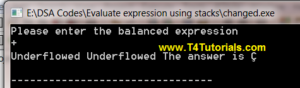