Printing Star by rows in C++ using inline functions
Here, I am sharing you a C++ program for printing the star by rows in C++ using inline functions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
#include<iostream> using namespace std; class patr { protected : int i,j,k,n; public : fun(); }; inline patr::fun() { cout<<"input No of rows : "; cin>>n; for(j=1 ; j<=n ; j++) { for(i=j ; i<n ; i++) { cout<<" "; } for(i=1 ; i<=j ; i++) { cout<<"*"; } cout<<"\n"; } } int main() { patr a; a.fun(); } |
Printing Star by rows in C++ using friend functions
Here, I am sharing with you a C++ program for printing the star by rows in C++ using the friend functions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
#include<iostream> using namespace std; class pat { protected : int i,j,r; public : input() { cout<<"input No of rows : "; cin>>r; } friend int show(pat); }; int show(pat a) { for(a.j=1 ; a.j<=a.r ; a.j++) { for(a.i=a.j ; a.i<a.r ; a.i++) { cout<<" "; } for(a.i=1 ; a.i<=a.j ; a.i++) { cout<<"*"; } cout<<"\n"; } } int main() { pat a; a.input(); show(a); } |
Printing Star by rows in C++ using constructor overloading
Here, I am sharing with you a C++ program for printing the star by rows in C++ by using constructor overloading.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 |
#include<iostream> using namespace std; class construct { protected : int i,j,k,n; public : construct(int n) { for(j=1 ; j<=n ; j++) { for(i=j ; i<n ; i++) { cout<<" "; } for(i=1 ; i<=j ; i++) { cout<<"*"; } cout<<"\n"; } } construct(int two,int three) { cout<<"\n\n1st patern is \n\n"; for(j=1 ; j<=two ; j++) { for(i=j ; i<two ; i++) { cout<<" "; } for(i=1 ; i<=j ; i++) { cout<<"*"; } cout<<"\n"; } cout<<"\n\n2nd patern is \n\n"; for(j=1 ; j<=three ; j++) { for(i=j ; i<three ; i++) { cout<<" "; } for(i=1 ; i<=j ; i++) { cout<<"*"; } cout<<"\n"; } } }; int main() { cout<<"\t\t\tThis Program Demonstrate The Single and"; cout<<"multiple paramter Constructor\n\n\n"; int option; cout<<"Enter 1 of Single parameter constructor \n"; cout<<"\nEnter 2 For Multiple Paramter constucor \n"; cout<<"\nEnter 1 or 2 : "; cin>>option; system("cls"); //this function is to clear the screen if(option ==1) { cout<<"\t\t\t-------You Have Slected Single Paramater"; cout<<"Constructor-------\n\n"; int n; cout<<"Enter Number of rows : "; cin>>n; construct a(n); } else if(option==2) { cout<<"\t\t\t-------You Have slected Multiple Paramater "; cout<<"Constructor-------\n\n"; int two,three; cout<<"\n\nEnter Number of rows for 1st patern : "; cin>>two; cout<<"\n\nEnter Number of rows for 2nd patern : "; cin>>three; construct a(two, three); } else { cout<<"Your Input in Wrong try Agin \n\n\n"; } } |
Printing Star by rows in C++ using the constructor
Here, I am sharing with you a C++ program for printing the star by rows in C++ by using the constructor.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
#include<iostream> using namespace std; class pat { protected : int i,j,r; public : pat() { cout<<"input No of rows : "; cin>>r; for(j=1 ; j<=r ; j++) { for(i=j ; i<r ; i++) { cout<<" "; } for(i=1 ; i<=j ; i++) { cout<<"*"; } cout<<"\n"; } } }; int main() { pat a; } |
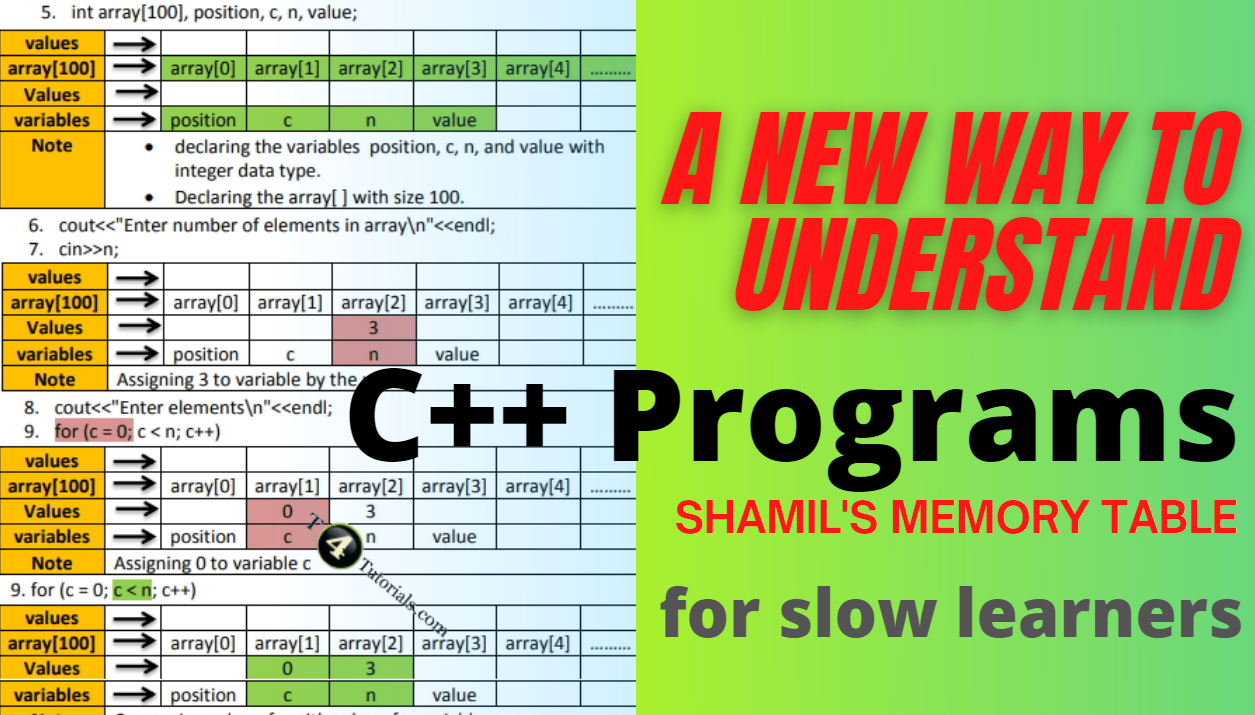