1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
#include <iostream> // Use iostream for C++ input/output using namespace std; // This allows us to use cout and endl without std:: prefix int main() { int a = 5; int b = 5; // a will increment first int c = ++a + 10; // b is used first int d = b++ + 10; // Output using cout cout << "Value of c: " << c << endl; // a is incremented first, then 10 is added cout << "Value of d: " << d << endl; // b is used first, then incremented cout << "Final value of b: " << b << endl; // b is now incremented return 0; } |
Prefix increment and post fix increment in C++ (C Plus Pls)
1 2 3 4 5 6 7 8 9 10 11 12 13 |
// Prefix increment and post fix increment in C++ (C Plus Pls) #include <iostream> using namespace std; int main() { int a,b,c,d; a=b=0; d=c=0; a=b++; //post fix c=++d; //pree fix cout<<"a="<<a<<endl<<"b="<<b<<endl; cout<<"c="<<c<<endl<<"d="<<d<<endl; } |
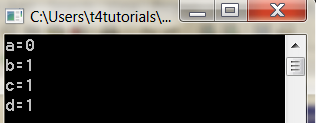
Prefix deccrement and post fix decrement in C++ (C Plus Pls)
1 2 3 4 5 6 7 8 9 10 11 12 13 |
// Prefix deccrement and post fix decrement in C++ (C Plus Pls) #include <iostream> using d=c=0;namespace std; int main() { int a,b,c,d; a=b=0; a=b--; //post fix decrement c= --d; //pree fix decrement cout<<"a="<<a<<endl<<"b="<<b<<endl; cout<<"c="<<c<<endl<<"d="<<d<<endl; } |
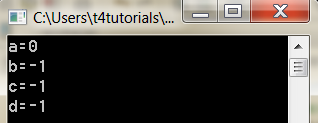
Prefix Increment and Postfix Increment in C++ using If else Statement
Lets begin with “Prefix Increment and Postfix Increment program in C++ using If else Statement”
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
#include<iostream> using namespace std; int main() { int a,b,c,d; a=b=0; c=d=0; if(a==0 && c==0) { a=b; b++; d++; c=d; } cout<<"a = "<<a<<endl<<"b = "<<b<<endl; cout<<"c = "<<c<<endl<<"d = "<<d<<endl; system("pause"); } |
Prefix Increment and Postfix Increment in C++ using for loop
Lets begin with “Prefix Increment and Postfix Increment program in C++ using for loop”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
#include<iostream> using namespace std; int main() { int a,b,c,d; a=b=0; c=d=0; for(int i=c; i<1; i++) { a=b++; c=++d; } cout<<"a = "<<a<<endl<<"b = "<<b<<endl; cout<<"c = "<<c<<endl<<"d = "<<d<<endl; system("pause"); } |
Prefix Increment and Postfix Increment in C++ using While loop
Lets begin with “Prefix Increment and Postfix Increment program in C++ using While loop”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 |
#include<iostream> using namespace std; int main() { int a,b,c,d; a=b=0; c=d=0; int i=c; while(i<1) { a=b++; c=++d; i++; } cout<<"a = "<<a<<endl<<"b = "<<b<<endl; cout<<"c = "<<c<<endl<<"d = "<<d<<endl; system("pause"); } |
Prefix Increment and Postfix Increment in C++ using Do While loop
Lets begin with “Prefix Increment and Postfix Increment program in C++ using Do While loop”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
#include<iostream> using namespace std; int main() { int a,b,c,d; a=b=0; c=d=0; int i=c; do { a=b++; c=++d; i++; } while(i<1); cout<<"a = "<<a<<endl<<"b = "<<b<<endl; cout<<"c = "<<c<<endl<<"d = "<<d<<endl; system("pause"); } |
Prefix Increment and Postfix Increment Program in C++ using the User Define Function
Lets begin with “Prefix Increment and Postfix Increment program in C++ using the user define function”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
#include<iostream> using namespace std; int fixes(int&,int&,int&,int&); int main() { int a,b,c,d; a=b=0; c=d=0; fixes(a,b,c,d); cout<<"a = "<<a<<endl<<"b = "<<b<<endl; cout<<"c = "<<c<<endl<<"d = "<<d<<endl; system("pause"); } int fixes(int &j, int &k, int &l, int &m) { j=k++; l=++m; } |