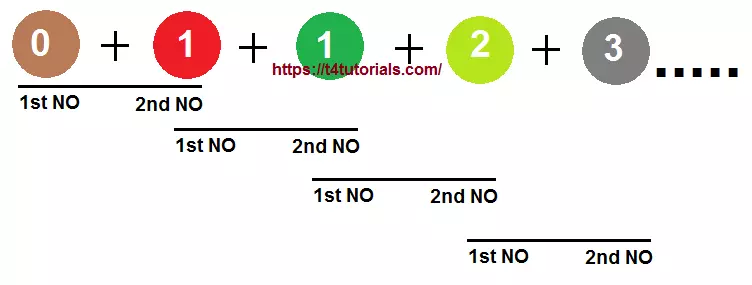
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
#include<iostream> using namespace std; class series { public: int a,b,t,j,num; public: int input() { a=0,b=1; cout<<"enter the number : "<<endl; cin>>num; cout<<"the fb series is : "<<a<<b; } int show() { for( j=3; j<=num; j++) { t=a+b; cout<<t; a=b; b=t; } } }; int main() { series obj; obj.input(); obj.show(); } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
#include<iostream> using namespace std; class series { protected: int a,b,t,j,num; }; class child:public series { public: int input() { a=0; b=1; cout<<"enter the number : "<<endl; cin>>num; cout<<"the fb series is : "<<a<<b; } public: int show() { for(j=3; j<=num; j++) { t=a+b; cout<<t; a=b; b=t; } } }; int main() { child obj; obj.input(); obj.show(); } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
#include<iostream> using namespace std; class series1 { protected: int a,b,num; }; class series2:public series1 { protected: int t,j; }; class series3:public series2 { public: int input() { a=0; b=1; cout<<"enter the number : "<<endl; cin>>num; cout<<"the fb series is : "<<a<<b; } public: int show() { for(j=3; j<=num; j++) { t=a+b; cout<<t; a=b; b=t; } } }; int main() { series3 obj; obj.input(); obj.show(); } |
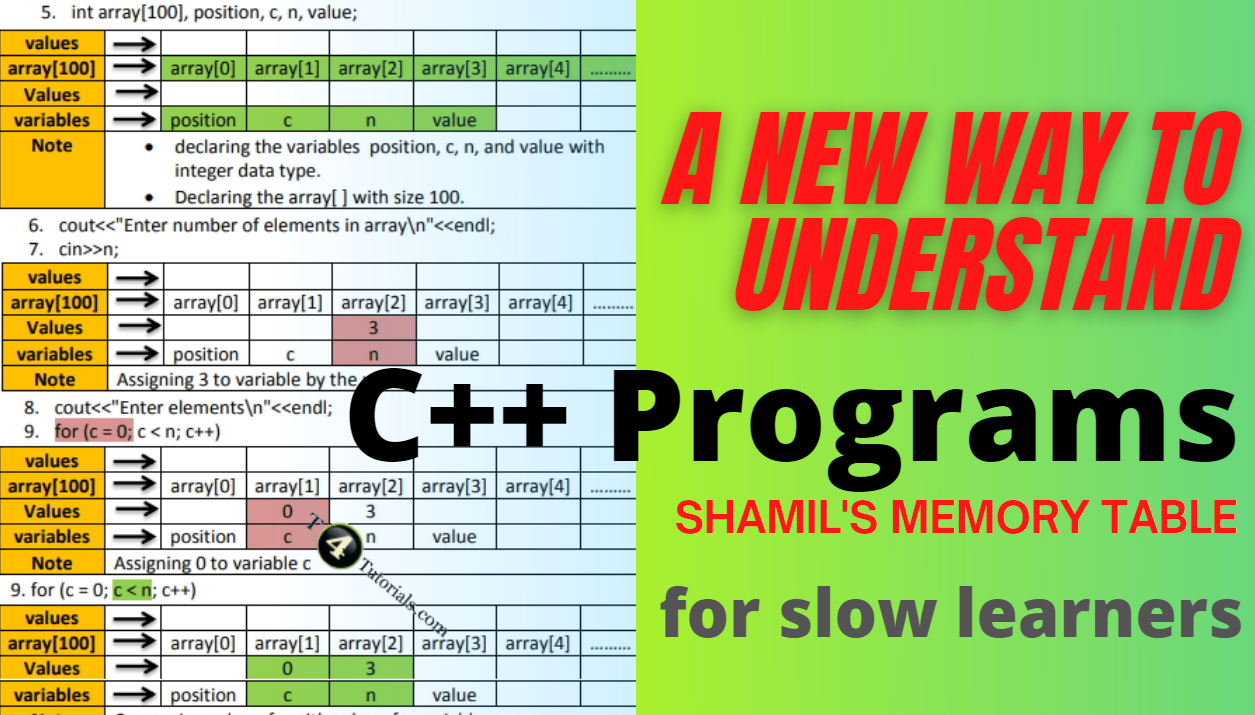