Arrays in C++
Arrays are used to store similar kind of data. Example: int marks[2 ]; Here marks is an array that can store two values.Examples of Array in C++ – Example 1
Program in which you “Enter No of times you want to Find Square Root”.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
#include<iostream> #include<Math.h> using namespace std; int main() { int HowManyTimes; cout<<"Enter No of HowManyTimes you want to Find Square Root "<<endl; cin>>HowManyTimes; float array[HowManyTimes]; for(int i=0;i<HowManyTimes;i++){ cout<<"Enter Value "<<i+1<<endl; cin>>array[i]; } for(int i=0;i<HowManyTimes;i++){ if(array[i] >=0 ) { cout<<"squareroot of "<<array[i]<<"is "<<sqrt(array[i])<<endl; } else { cout<<"complex root"<<endl; } } return 0; } |
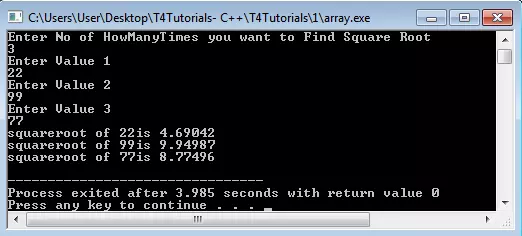
Examples of Array in C++ – Example 2
Program to insert an element in an array at a specific position.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 |
#include<iostream> using namespace std; int main() { int ArrayToStore[100]; int size; int place; int value; cout<<"Please type the number of values:"<<endl; cin>>size; cout<<"Enter values:"<<endl; int i=0; do { cin>>ArrayToStore[i]; i++; } while(i<size); cout<<"Current values are:"<<endl; for(int i=0; i<size; i++) { cout<<ArrayToStore[i]<<" "; } cout<<endl<<"please type place where you want to insert value:"<<endl; cin>>place; cout<<"Please Enter the new value:"<<endl; cin>>value; for(int i=size; i>=place; i--) { ArrayToStore[i]=ArrayToStore[i-1]; } ArrayToStore[place-1]=value; cout<<"The ArrayToStore after new values:"<<endl; i=0; while(i<size+1) { cout<<ArrayToStore[i]<<" "; i++; } } |
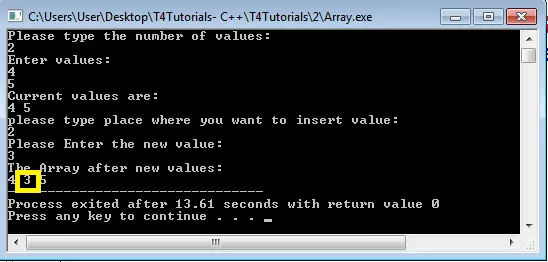
Examples of Array in C++ – Example 3
Program to combine two string in C++.
1 2 3 4 5 6 7 8 9 10 11 12 |
#include<iostream> #include<string.h> using namespace std; int main() { char a[10]="T4",b[10]="Tutorials"; cout<<"1st string = "<<a<<endl; cout<<"2nd string = "<<b<<endl; strcat(a,b); cout<<"The combined string is = "<<a; } |
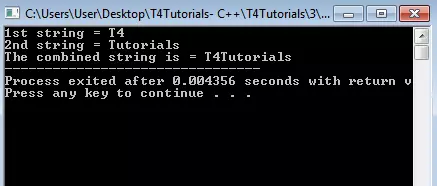