Program to convert a temperature in Celsius to Fahrenheit in c++ and C with the flowchart.
Given F = 9 5 C + 32, the conversion formula for Fahrenheit to Celsius, solve for C.
2.
Flowchart of a program to convert a temperature in Celsius to Fahrenheit
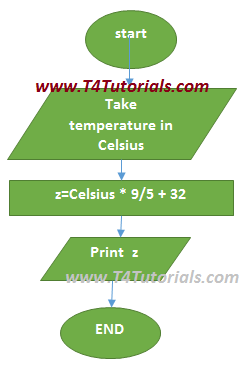
Program to convert a temperature in Celsius to Fahrenheit in c++
1 2 3 4 5 6 7 8 9 10 11 12 13 |
#include<iostream> #include<conio.h> using namespace std; int main() { float C; float F; cout<<"input temperature in Celsius is= "; cin>>C; F= C * 9/5 + 32; cout<<"Temperature in Fahrenheit is= "<<F; getch(); } |
Output
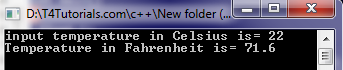
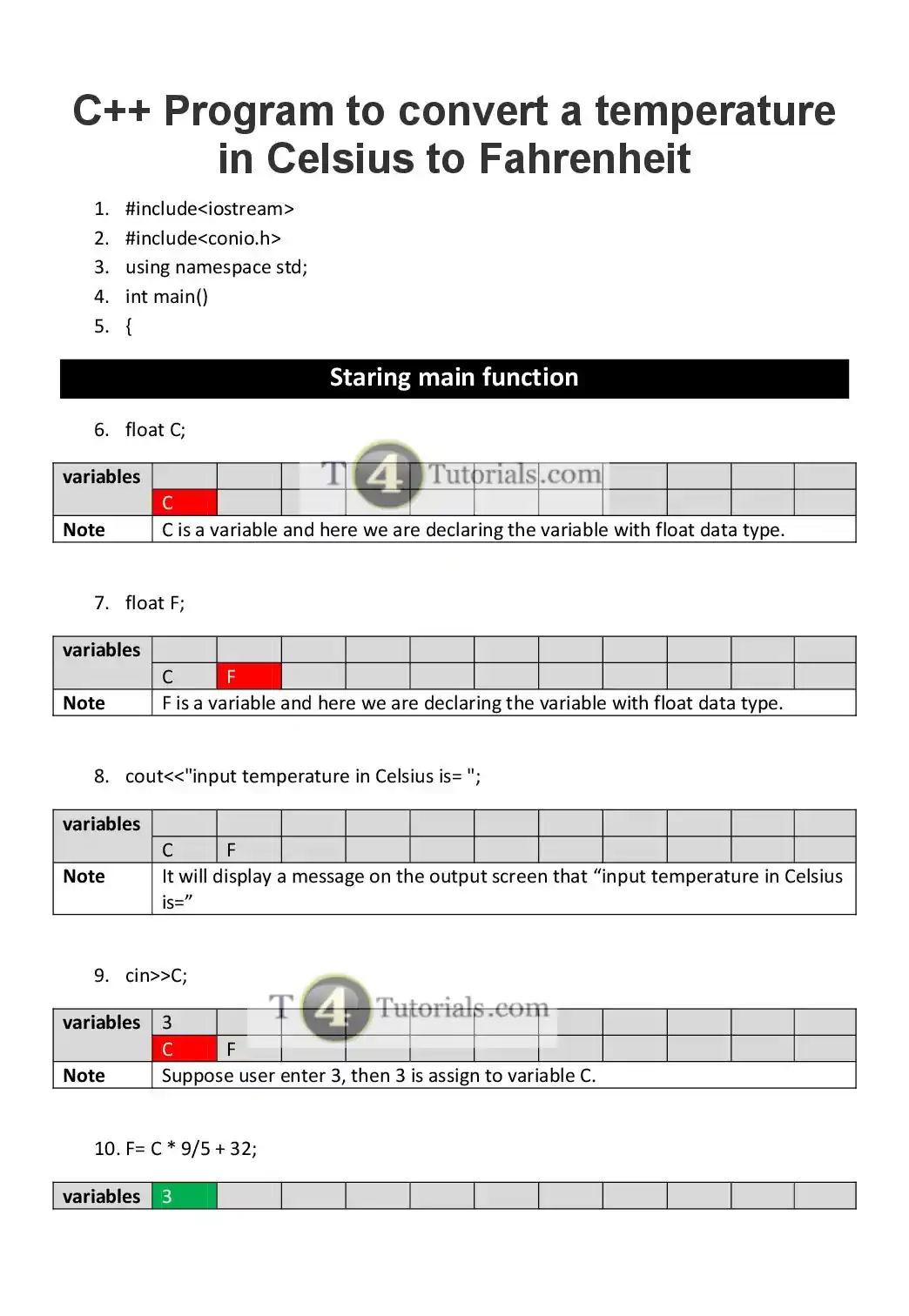
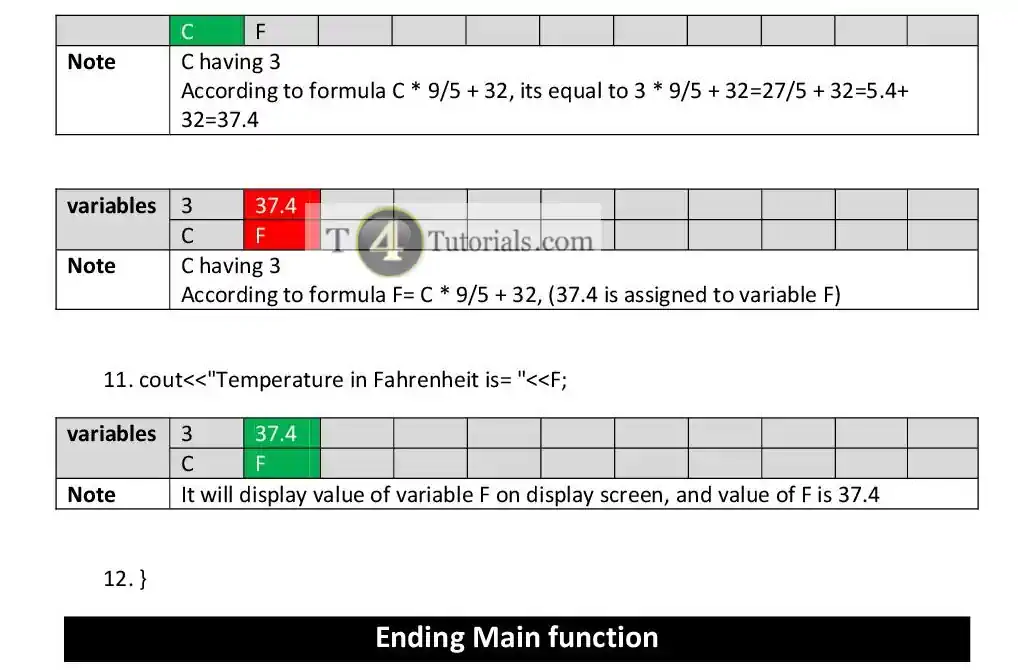
Program to convert a temperature in Celsius to Fahrenheit in C
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
#include <stdio.h> int main() { float C; float F; printf("input temperature in Celsius: "); scanf("%f", &C); F = (C * 9 / 5) + 32; printf("%.2f Celsius = %.2f Fahrenheit=", C, F); return 0; } |
Output
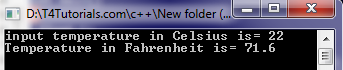
Celsius to Fahrenheit C++ while loop
1 2 3 4 5 6 7 8 9 10 11 12 |
#include <iostream> using namespace std; int main() { float T4Tutorials_Celsius; float T4Tutorials_fahrenheit; cout << " Celsius to fahrenheit conversion." << endl; while (cin >> T4Tutorials_Celsius) { // (1) T4Tutorials_fahrenheit = 1.8 * T4Tutorials_Celsius + 32; cout << " fahrenheit = " << T4Tutorials_fahrenheit << endl; } return 0; } |
Celsius to Fahrenheit C++ for loop
Writ a celsius C++ program to show the Fahrenheit table.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
#include <iostream> #include <iomanip> using namespace std; int main() { float T4Tutorials_fahrenheit = 0; cout << endl; cout << "*****************\n"; cout << "Celsius\t\tFahrenheit" << endl; cout << "*****************\n"; cout << setprecision(1) << fixed << right; cout.fill('0'); for (int T4Tutorials_Celsius = 0; T4Tutorials_Celsius <= 20; T4Tutorials_Celsius++) { // Calculate Fahrenheit T4Tutorials_fahrenheit = ((9.0 / 5) * T4Tutorials_Celsius) + 32; cout << setw(2) << T4Tutorials_Celsius << "\t\t" << T4Tutorials_fahrenheit << endl; } cout << "*****************\n"; return 0; } |