C++ program to print hollow right triangle star pattern with class objects C++.
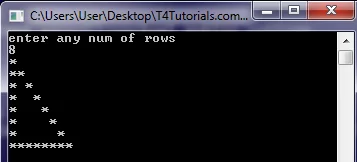
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 |
//program to print hollow right triangle star pattren //simple program #include<iostream> using namespace std; class pattren { private: int i,j,rows; public: int number() { cout<<"enter any num of rows"<<endl; cin>>rows; for(i=1; i<=rows; i++) { for(j=1; j<=i; j++) { if(j==1 || j==i || i==rows) { cout<<"*"; } else { cout<<" "; } } cout<<endl; } } }; int main() { pattren f; f.number(); } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
//program to print hollow right triangle star pattren //single inheritence program #include<iostream> using namespace std; class pattren { protected: int i,j,rows; }; class child:public pattren { public: int number() { cout<<"enter any num of rows"<<endl; cin>>rows; for(i=1; i<=rows; i++) { for(j=1; j<=i; j++) { if(j==1 || j==i || i==rows) { cout<<"*"; } else { cout<<" "; } } cout<<endl; } } }; int main() { child f; f.number(); } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
//program to print hollow right triangle star pattren //multiple inheritence program #include<iostream> using namespace std; class pattren { protected: int i,j; }; class pattren2 { protected: int rows; }; class child:public pattren,public pattren2 { public: int number() { cout<<"enter any num of rows"<<endl; cin>>rows; for(i=1; i<=rows; i++) { for(j=1; j<=i; j++) { if(j==1 || j==i || i==rows) { cout<<"*"; } else { cout<<" "; } } cout<<endl; } } }; int main() { child f; f.number(); } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
//program to print hollow right triangle star pattren //multi level inheritence program #include<iostream> using namespace std; class pattren { protected: int i,j; }; class pattren2:public pattren { protected: int rows; }; class child:public pattren2 { public: int number() { cout<<"enter any num of rows"<<endl; cin>>rows; for(i=1; i<=rows; i++) { for(j=1; j<=i; j++) { if(j==1 || j==i || i==rows) { cout<<"*"; } else { cout<<" "; } } cout<<endl; } } }; int main() { child f; f.number(); } |