Delete Array Elements using Constructor Destructor and Classes Inheritance in OOP – C++
In this tutorial, we will code the Program to Delete the Array Elements with OOP Classes.
Delete Array Elements Using Classes
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 |
#include<iostream> using namespace std; class deleteetion { public: int DELETE() { int MyArray[22], size, i, delete, counter=0; cout<<"Plz enter MyArrayay Size : "; cin>>size; cout<<"Plz enter MyArrayary element :"; for (i=0; i<size; i++) { cin>>MyArray[i]; } cout<<"Plz enter The element to deleteete:"; cin>> delete; for(i=0; i<size; i++) { if(MyArray[i]==delete) { for (int j=i; j<(size-1); j++) { MyArray[j] = MyArray[j+1]; } counter++; } } if(counter==0) { cout<<"Element Not Found...!!!"; } else { cout<<"elemet deleteeted successfully..!!"<<endl; cout<<"Now The new MyArrayay Is :"<<endl; for(i=0; i<(size-1); i++) { cout<<MyArray[i]<<" "; } } } }; int main() { ; deleteetion detleteMyArrayaynumer; detleteMyArrayaynumer.DELETE(); } |
Output
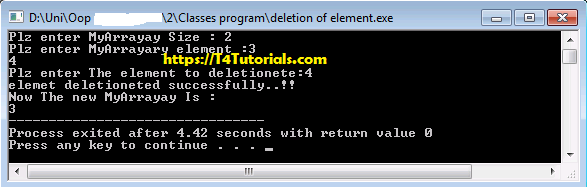
Delete Array Elements Using Constructor
Below is the code for deleting the array elements using the constructor.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 |
#include<iostream> using namespace std; class DELETION { public: DELETION() { int arr[50], size, i, deletion, count=0; cout<<"enter Array Size : "; cin>>size; cout<<"enter arrary element :"; for (i=0; i<size; i++) { cin>>arr[i]; } cout<<"enter The element to deletionete:"; cin>> deletion; for(i=0; i<size; i++) { if(arr[i]==deletion) { for (int j=i; j<(size-1); j++) { arr[j] = arr[j+1]; } count++; } } if(count==0) { cout<<"Element Not Found."; } else { cout<<"elemet deletioneted successfully."<<endl; cout<<"Now The new Array Is :"<<endl; for(i=0; i<(size-1); i++) { cout<<arr[i]<<" "; } } } }; int main() { DELETION A; } |
Output
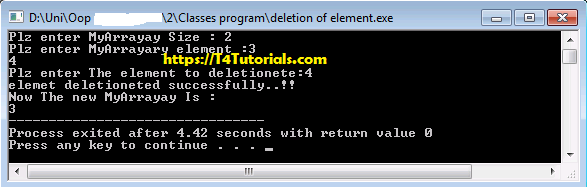
Delete Array Elements Using Destructor
Below is the code for deleting the array elements using the destructor.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 |
#include<iostream> using namespace std; class DELETION { public: DELETION() { int arr[50], size, i, deletion, count=0; cout<<"enter Array Size : "; cin>>size; cout<<"enter arrary element :"; for (i=0; i<size; i++) { cin>>arr[i]; } cout<<"enter The element to deletionete:"; cin>> deletion; for(i=0; i<size; i++) { if(arr[i]==deletion) { for (int j=i; j<(size-1); j++) { arr[j] = arr[j+1]; } count++; } } if(count==0) { cout<<"Element Not Found...!!!"; } else { cout<<"elemet deletioneted successfully..!!"<<endl; cout<<"Now The new Array Is :"<<endl; for(i=0; i<(size-1); i++) { cout<<arr[i]<<" "; } } } ~DELETION() { int arr[50], size, i, deletion, count=0; cout<<"enter Array Size : "; cin>>size; cout<<"enter arrary element :"; for (i=0; i<size; i++) { cin>>arr[i]; } cout<<"enter The element to deletionete:"; cin>> deletion; for(i=0; i<size; i++) { if(arr[i]==deletion) { for (int j=i; j<(size-1); j++) { arr[j] = arr[j+1]; } count++; } } if(count==0) { cout<<"Element Not Found."; } else { cout<<"elemet deletioneted successfully."<<endl; cout<<"Now The new Array Is :"<<endl; for(i=0; i<(size-1); i++) { cout<<arr[i]<<" "; } } } }; int main() { DELETION A; } |
Output
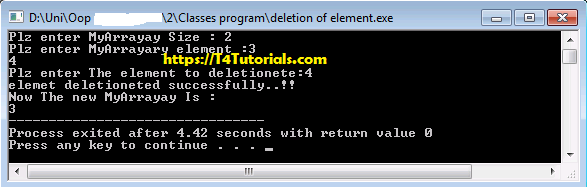
Delete Array Elements Using Inheritance
Below is the code for deleting the array elements using the inheritance.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 |
#include<iostream> using namespace std; class deletion { protected: int arr[50], size, i, del, count=0; }; class deleting : public deletion { public: int DEL() { int arr[50], size, i, del, count=0; cout<<"Please Enter Array Size : "; cin>>size; cout<<"Please Enter arrary element :"; for (i=0; i<size; i++) { cin>>arr[i]; } cout<<"Please Enter The element to delete:"; cin>> del; for(i=0; i<size; i++) { if(arr[i]==del) { for (int j=i; j<(size-1); j++) { arr[j] = arr[j+1]; } count++; } } if(count==0) { cout<<"Element Not Found...!!!"; } else { cout<<"elemet deleted successfully..!!"<<endl; cout<<"Now The new Array Is :"<<endl; for(i=0; i<(size-1); i++) { cout<<arr[i]<<" "; } } } }; int main() { deleting DetleteArrayNumber; DetleteArrayNumber.DEL(); } |
Output
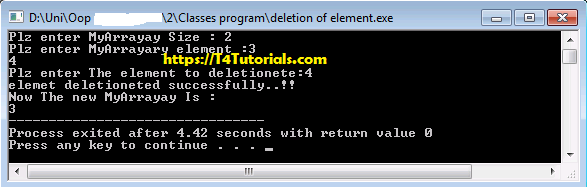